Computer Applications
Write a program to input a number in the range 10 to 100 and check if it is a prime number.
Java
Java Iterative Stmts
11 Likes
Answer
import java.util.Scanner;
public class KboatPrime
{
public static void main(String args[]) {
System.out.print("Enter a number between 10 to 100 : ");
Scanner in = new Scanner(System.in);
int num = in.nextInt();
int c = 0;
if(num < 10 || num > 100)
System.out.println("Number out of range");
else {
for (int i = 1; i <= num; i++) {
if (num % i == 0)
c++;
}
if (c == 2)
System.out.println("Prime number");
else
System.out.println("Not a prime number");
}
}
}
Variable Description Table
Program Explanation
Output
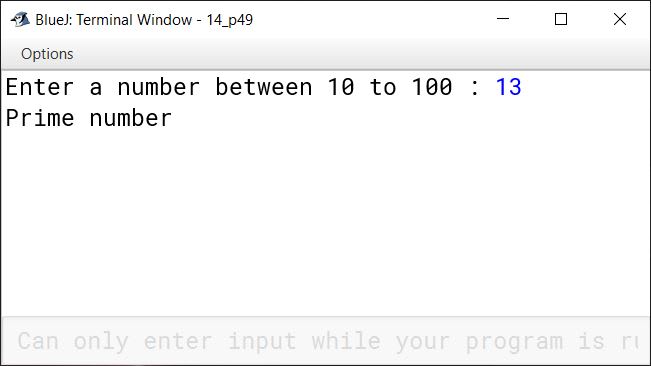
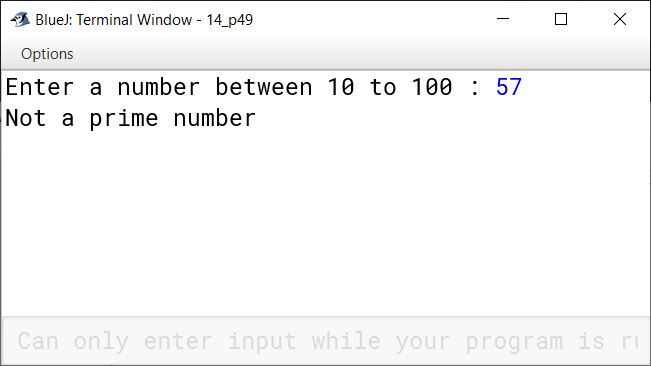
Answered By
3 Likes
Related Questions
Write a program to print following series of numbers: 2, 5, 8, 11, 14….
Write a program to print Fibonacci series : 0, 1, 1, 2, 3, 5, 8….
Write a program that prints the squares of 10 even numbers in the range 10 .. 100.
Palindrome Number in Java: Write a program to accept a number from the user and check whether it is a Palindrome number or not. A number is a Palindrome which when reads in reverse order is same as in the right order.
Sample Input: 242
Sample Output: A Palindrome numberSample Input: 467
Sample Output: Not a Palindrome number