Computer Applications
Palindrome Number in Java: Write a program to accept a number from the user and check whether it is a Palindrome number or not. A number is a Palindrome which when reads in reverse order is same as in the right order.
Sample Input: 242
Sample Output: A Palindrome number
Sample Input: 467
Sample Output: Not a Palindrome number
Java
Java Iterative Stmts
223 Likes
Answer
import java.util.Scanner;
public class KboatPalindromeNumber
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter the number: ");
int num = in.nextInt();
int copyNum = num;
int revNum = 0;
while(copyNum != 0) {
int digit = copyNum % 10;
copyNum /= 10;
revNum = revNum * 10 + digit;
}
if (revNum == num)
System.out.println("A Palindrome number");
else
System.out.println("Not a Palindrome number");
}
}
Variable Description Table
Program Explanation
Output
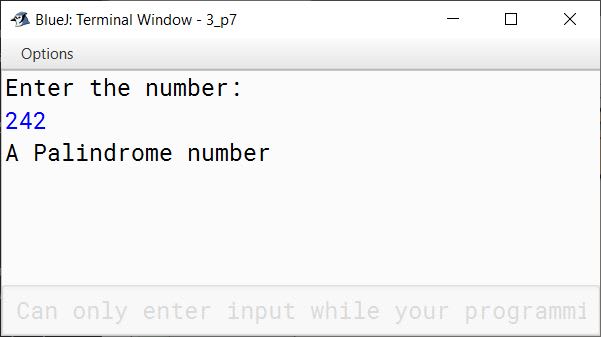
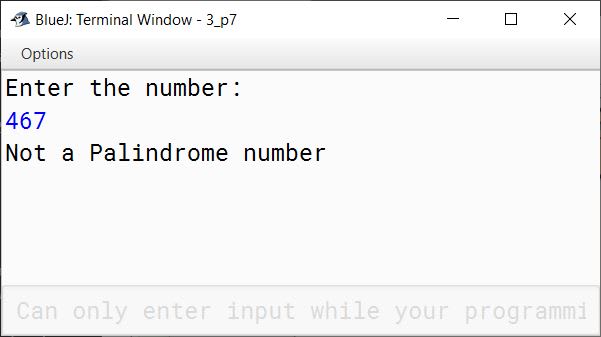
Answered By
96 Likes
Related Questions
Write a program to accept marks in Physics, Chemistry and Biology. The program calculates the average and displays the stream accordingly:
Average Marks Stream 80% and above Computer Science 60% or more but less than 80% Bio-Science 40% or more but less than 60% Commerce The Greatest Common Divisor (GCD) of two integers is calculated by the continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process repeats unless the remainder reaches to zero. The last divisor results in GCD.
Sample Input: 45, 20
Sample Output: GCD=5Write a program to find the sum of the given series:
S = 1 + (1*2) + (1*2*3) + --------- to 10 terms.
Write a program to find the sum of the given series:
S = (2/3) + (4/5) + (8/7) + --------- to n terms.