Computer Applications
Write a program to accept marks in Physics, Chemistry and Biology. The program calculates the average and displays the stream accordingly:
Average Marks | Stream |
---|---|
80% and above | Computer Science |
60% or more but less than 80% | Bio-Science |
40% or more but less than 60% | Commerce |
Java
Java Conditional Stmts
69 Likes
Answer
import java.util.Scanner;
public class KboatAvgNStream
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter marks in Physics: ");
int phy = in.nextInt();
System.out.print("Enter marks in Chemistry: ");
int chem = in.nextInt();
System.out.print("Enter marks in Biology: ");
int bio = in.nextInt();
double avg = (phy + chem + bio) / 3.0;
if (avg < 40)
System.out.println("No stream");
else if (avg < 60)
System.out.println("Commerce");
else if (avg < 80)
System.out.println("Bio-Science");
else
System.out.println("Computer Science");
}
}
Variable Description Table
Program Explanation
Output
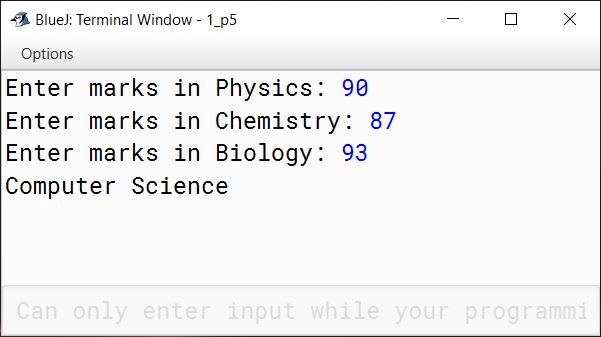
Answered By
30 Likes
Related Questions
Write a program to find the sum of the given series:
S = 1 + (1*2) + (1*2*3) + --------- to 10 terms.
The Greatest Common Divisor (GCD) of two integers is calculated by the continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process repeats unless the remainder reaches to zero. The last divisor results in GCD.
Sample Input: 45, 20
Sample Output: GCD=5Write a program to find the sum of the given series:
S = (2/3) + (4/5) + (8/7) + --------- to n terms.
Palindrome Number in Java: Write a program to accept a number from the user and check whether it is a Palindrome number or not. A number is a Palindrome which when reads in reverse order is same as in the right order.
Sample Input: 242
Sample Output: A Palindrome numberSample Input: 467
Sample Output: Not a Palindrome number