Computer Applications
Write a program to input a number. Calculate its square root and cube root. Finally, display the result by rounding it off.
Sample Input: 5
Square root of 5= 2.2360679
Rounded form= 2
Cube root of 5 = 1.7099759
Rounded form= 2
Java
Java Math Lib Methods
77 Likes
Answer
import java.util.Scanner;
public class KboatRoots
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the number: ");
int num = in.nextInt();
double sqrtValue = Math.sqrt(num);
double cbrtValue = Math.cbrt(num);
long roundSqrt = Math.round(sqrtValue);
long roundCbrt = Math.round(cbrtValue);
System.out.println("Square root of " + num + " = " + sqrtValue);
System.out.println("Rounded form = " + roundSqrt);
System.out.println("Cube root of " + num + " = " + cbrtValue);
System.out.println("Rounded form = " + roundCbrt);
}
}
Variable Description Table
Program Explanation
Output
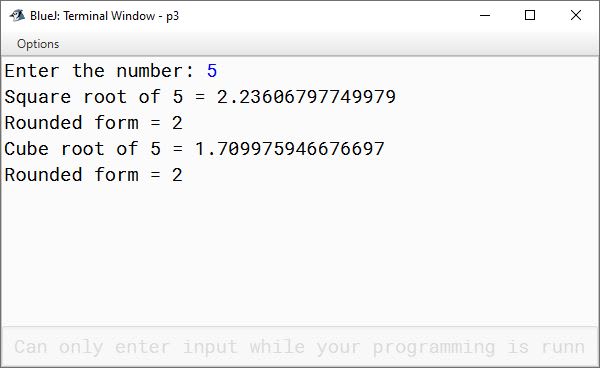
Answered By
34 Likes
Related Questions
Write a program to calculate the value of the given expression:
1/a2 + 2/b2 + 3/c2
Take the values of a, b and c as input from the console. Finally, display the result of the expression to its nearest whole number.For every natural number m>1; 2m, m2-1 and m2+1 form a Pythagorean triplet. Write a program to input the value of 'm' through console to display a 'Pythagorean Triplet'.
Sample Input: 3
Then 2m=6, m2-1=8 and m2+1=10
Thus 6, 8, 10 form a 'Pythagorean Triplet'.The volume of a sphere is calculated by using formula:
v = (4/3)*(22/7)*r3Write a program to calculate the radius of a sphere by taking its volume as an input.
Hint: radius = ∛(volume * (3/4) * (7/22))
A trigonometrical expression is given as:
(Tan A - Tan B) / (1 + Tan A * Tan B)Write a program to calculate the value of the given expression by taking the values of angles A and B (in degrees) as input.
Hint: radian= (22 / (7 * 180)) * degree