Computer Applications
A trigonometrical expression is given as:
(Tan A - Tan B) / (1 + Tan A * Tan B)
Write a program to calculate the value of the given expression by taking the values of angles A and B (in degrees) as input.
Hint: radian= (22 / (7 * 180)) * degree
Java
Java Math Lib Methods
37 Likes
Answer
import java.util.Scanner;
public class KboatTrigExp
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter angle A in degrees: ");
double angleADeg = in.nextDouble();
System.out.print("Enter angle B in degrees: ");
double angleBDeg = in.nextDouble();
double angleARad = (22 * angleADeg) / (7 * 180);
double angleBRad = (22 * angleBDeg) / (7 * 180);
double numerator = Math.tan(angleARad) - Math.tan(angleBRad);
double demoninator = 1 + Math.tan(angleARad) * Math.tan(angleBRad);
double trigExp = numerator / demoninator;
System.out.println("tan(A - B) = " + trigExp);
}
}
Variable Description Table
Program Explanation
Output
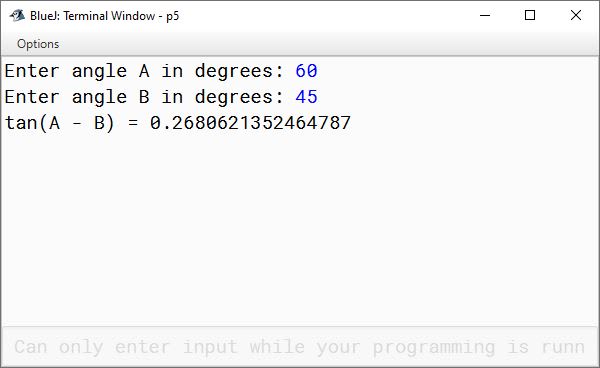
Answered By
13 Likes
Related Questions
The sum of first n odd natural numbers can be calculated as n2, where n is the number of odd natural.
For example,
Sum = 1 + 3 + 5 + 7; number of odd natural = 4
Therefore, Sum = 42 = 16
Write a program to input number of odd natural terms and display sum of the given series:
(a) 1 + 3 + 5 + ………………. + 29 + 31
(b) 1 + 3 + 5 + 7 + ………………. + 47 + 49The standard form of quadratic equation is represented as:
ax2 + bx + c = 0where d= b2 - 4ac, known as 'Discriminant' of the equation.
Write a program to input the values of a, b and c. Calculate the value of discriminant and display the output to the nearest whole number.
The volume of a sphere is calculated by using formula:
v = (4/3)*(22/7)*r3Write a program to calculate the radius of a sphere by taking its volume as an input.
Hint: radius = ∛(volume * (3/4) * (7/22))
Write a program to input a number. Calculate its square root and cube root. Finally, display the result by rounding it off.
Sample Input: 5
Square root of 5= 2.2360679
Rounded form= 2
Cube root of 5 = 1.7099759
Rounded form= 2