Computer Applications
Write a program to assign a full path and file name as given below. Using library functions, extract and output the file path, file name and file extension separately as shown.
Input
C:\Users\admin\Pictures\flower.jpg
Output
Path: C:\Users\admin\Pictures\
File name: flower
Extension: jpg
Java
Java String Handling
ICSE 2014
67 Likes
Answer
import java.util.Scanner;
public class KboatFilepathSplit
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter full path: ");
String filepath = in.next();
char pathSep = '\\';
char dotSep = '.';
int pathSepIdx = filepath.lastIndexOf(pathSep);
System.out.println("Path:\t\t" + filepath.substring(0, pathSepIdx));
int dotIdx = filepath.lastIndexOf(dotSep);
System.out.println("File Name:\t" + filepath.substring(pathSepIdx + 1, dotIdx));
System.out.println("Extension:\t" + filepath.substring(dotIdx + 1));
}
}
Output
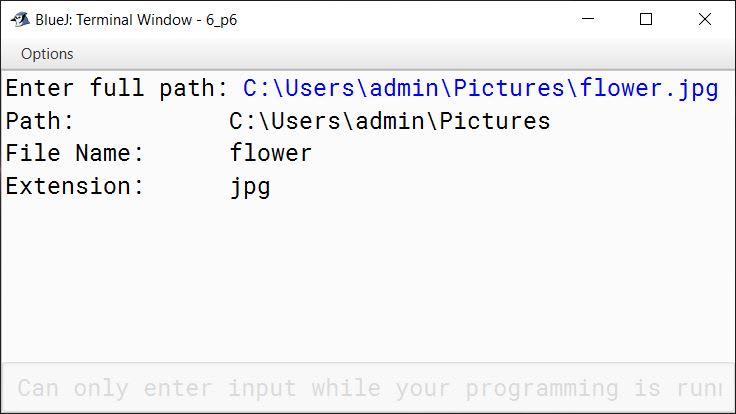
Answered By
25 Likes
Related Questions
Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Define a class to accept the gmail id and check for its validity.
A gmail id is valid only if it has:
→ @
→ .(dot)
→ gmail
→ com
Example:
icse2024@gmail.com
is a valid gmail idWrite a program in Java to enter any sentence. Also ask the user to enter a word. Print the number of times the word entered is present in the sentence. If the word is not present in the sentence, then print an appropriate message.