Computer Applications
Write a program to assign a full path and file name as given below. Using library functions, extract and output the file path, file name and file extension separately as shown.
Input
C:\Users\admin\Pictures\flower.jpg
Output
Path: C:\Users\admin\Pictures\
File name: flower
Extension: jpg
Java
Java String Handling
ICSE 2014
67 Likes
Answer
import java.util.Scanner;
public class KboatFilepathSplit
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter full path: ");
String filepath = in.next();
char pathSep = '\\';
char dotSep = '.';
int pathSepIdx = filepath.lastIndexOf(pathSep);
System.out.println("Path:\t\t" + filepath.substring(0, pathSepIdx));
int dotIdx = filepath.lastIndexOf(dotSep);
System.out.println("File Name:\t" + filepath.substring(pathSepIdx + 1, dotIdx));
System.out.println("Extension:\t" + filepath.substring(dotIdx + 1));
}
}
Output
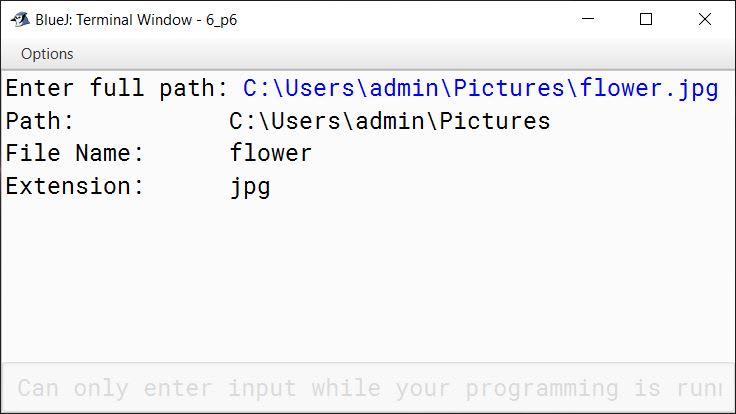
Answered By
25 Likes
Related Questions
Which of the following returns a value greater than or equal to 0?
A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Write a Java program to enter any sentence and convert the sentence to uppercase. Print only those words of the sentence whose first and last letters are the same.
Write the output of the following String methods:
String x= "Galaxy", y= "Games";
(a) System.out.println(x.charAt(0)==y.charAt(0));
(b) System.out.println(x.compareTo(y));