Computer Applications
Write a Java program to enter any sentence and convert the sentence to uppercase. Print only those words of the sentence whose first and last letters are the same.
Java
Java String Handling
4 Likes
Answer
import java.util.Scanner;
public class KboatString
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence: ");
String str = in.nextLine();
str = str.toUpperCase();
str += " ";
int len = str.length();
String word = "";
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ') {
char first = word.charAt(0);
char last = word.charAt(word.length() - 1);
if (first == last) {
System.out.println(word);
}
word = "";
}
else {
word += ch;
}
}
}
}
Output
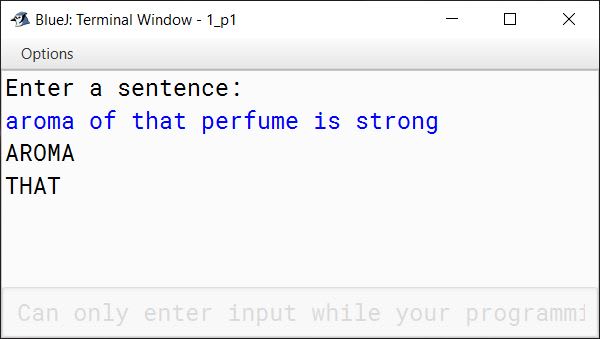
Answered By
1 Like
Related Questions
The output of the statement "CONCENTRATION".indexOf('T') is:
- 9
- 7
- 6
- (-1)
The output of the statement "talent".compareTo("genius") is:
- 11
- –11
- 0
- 13
A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Define a class to accept a string and convert the same to uppercase, create and display the new string by replacing each vowel by immediate next character and every consonant by the previous character. The other characters remain the same.
Example:
Input : #IMAGINATION@2024
Output : #JLBFJMBSJPM@2024