Computer Applications
Write a program to accept a string. Convert the string into upper case letters. Count and output the number of double letter sequences that exist in the string.
Sample Input: "SHE WAS FEEDING THE LITTLE RABBIT WITH AN APPLE"
Sample Output: 4
Java
Java String Handling
ICSE 2012
201 Likes
Answer
import java.util.Scanner;
public class KboatLetterSeq
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter string: ");
String s = in.nextLine();
String str = s.toUpperCase();
int count = 0;
int len = str.length();
for (int i = 0; i < len - 1; i++) {
if (str.charAt(i) == str.charAt(i + 1))
count++;
}
System.out.println("Double Letter Sequence Count = " + count);
}
}
Variable Description Table
Program Explanation
Output
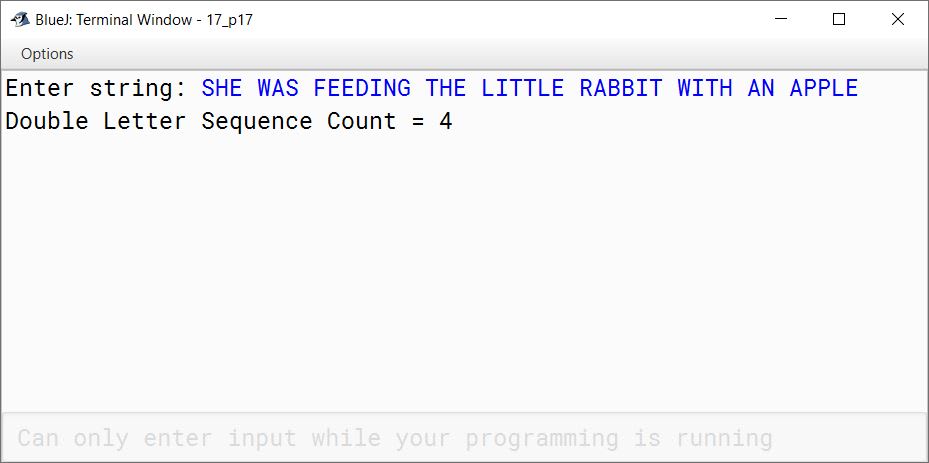
Answered By
81 Likes
Related Questions
Write a program to input a sentence. Count and display the frequency of each letter of the sentence in alphabetical order.
Sample Input: COMPUTER APPLICATIONS
Sample Output:Character Frequency Character Frequency A 2 O 2 C 2 P 3 I 1 R 1 L 2 S 1 M 1 T 2 N 1 U 1 A 'Happy Word' is defined as:
Take a word and calculate the word’s value based on position of the letters in English alphabet. On the basis of word’s value, find the sum of the squares of its digits. Repeat the process with the resultant number until the number equals 1 (one). If the number ends with 1 then the word is called a 'Happy Word'.
Write a program to input a word and check whether it a ‘Happy Word’ or not. The program displays a message accordingly.
Sample Input: VAT
Place value of V = 22, A= 1, T = 20
[Hint: A = 1, B = 2, ----------, Z = 26]Solution:
22120 ⇒ 22 + 22 + 12 + 22 + 02 = 13
⇒ 12 + 32 = 10
⇒ 12 + 02 = 1Sample Output: A Happy Word
Special words are those words which start and end with the same letter.
Example: EXISTENCE, COMIC, WINDOW
Palindrome words are those words which read the same from left to right and vice-versa.
Example: MALYALAM, MADAM, LEVEL, ROTATOR, CIVIC
All palindromes are special words but all special words are not palindromes.Write a program to accept a word. Check and display whether the word is a palindrome or only a special word or none of them.
Write a program to input a sentence. Convert the sentence into upper case letters. Display the words along with frequency of the words which have at least a pair of consecutive letters.
Sample Input: MODEM IS AN ELECTRONIC DEVICE
Sample Output:
MODEM
DEVICE
Number of words containing consecutive letters: 2