Computer Applications
Special words are those words which start and end with the same letter.
Example: EXISTENCE, COMIC, WINDOW
Palindrome words are those words which read the same from left to right and vice-versa.
Example: MALYALAM, MADAM, LEVEL, ROTATOR, CIVIC
All palindromes are special words but all special words are not palindromes.
Write a program to accept a word. Check and display whether the word is a palindrome or only a special word or none of them.
Java
Java String Handling
ICSE 2016
96 Likes
Answer
import java.util.Scanner;
public class KboatSpecialPalindrome
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a word: ");
String str = in.next();
str = str.toUpperCase();
int len = str.length();
if (str.charAt(0) == str.charAt(len - 1)) {
boolean isPalin = true;
for (int i = 1; i < len / 2; i++) {
if (str.charAt(i) != str.charAt(len - 1 - i)) {
isPalin = false;
break;
}
}
if (isPalin) {
System.out.println("Palindrome");
}
else {
System.out.println("Special");
}
}
else {
System.out.println("Neither Special nor Palindrome");
}
}
}
Variable Description Table
Program Explanation
Output
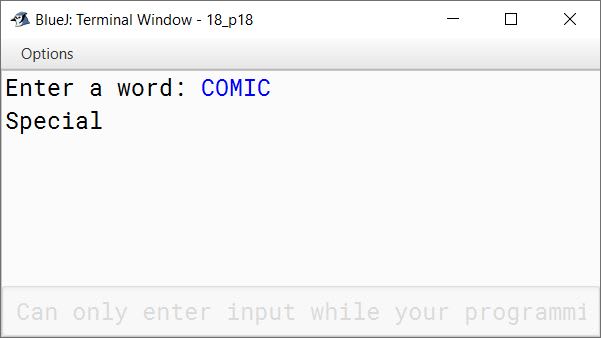
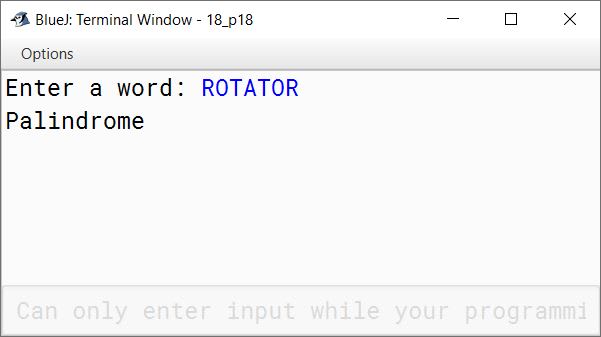
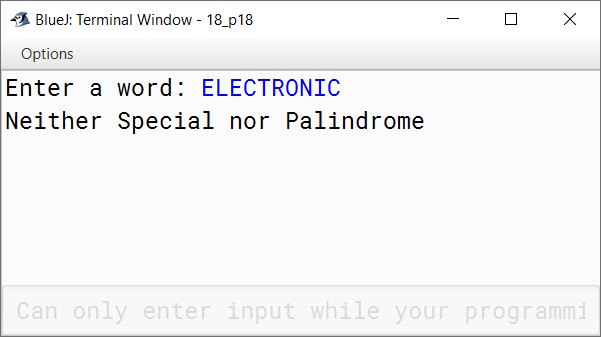
Answered By
23 Likes
Related Questions
Write a program to input a sentence. Convert the sentence into upper case letters. Display the words along with frequency of the words which have at least a pair of consecutive letters.
Sample Input: MODEM IS AN ELECTRONIC DEVICE
Sample Output:
MODEM
DEVICE
Number of words containing consecutive letters: 2Write a program to accept a word (say, BLUEJ) and display the pattern:
B L U E J
B L U E
B L U
B L
BWrite a program to input a sentence. Count and display the frequency of each letter of the sentence in alphabetical order.
Sample Input: COMPUTER APPLICATIONS
Sample Output:Character Frequency Character Frequency A 2 O 2 C 2 P 3 I 1 R 1 L 2 S 1 M 1 T 2 N 1 U 1 Write a program to accept a string. Convert the string into upper case letters. Count and output the number of double letter sequences that exist in the string.
Sample Input: "SHE WAS FEEDING THE LITTLE RABBIT WITH AN APPLE"
Sample Output: 4