Computer Science
Write a program to accept a set of n integers (where n > 0) in a single dimensional array. Arrange the elements of the array such that the lowest number appears in the centre of the array, next lower number in the right cell of the centre, next lower in the left cell of the centre and so on… . The process will stop when the highest number will set in its appropriate cell. Finally, display the array elements.(Pendulum Arrangement of Array)
Assume that the memory space is less. Hence, you don't need to create extra array for the aforesaid task.
Example:
Input: 1 2 3 4 5
Output: 5 3 1 2 4
Input: 11 12 31 14 5
Output: 31 12 5 11 14
Java
Java Arrays
16 Likes
Answer
import java.util.Scanner;
public class KboatSDAArrange
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter n: ");
int n = in.nextInt();
if (n <= 0) {
System.out.println("Invalid Input! n should be greater than 0.");
return;
}
int arr[] = new int[n];
System.out.println("Enter array elements:");
for (int i = 0; i < n; i++) {
arr[i] = in.nextInt();
}
/*
* Steps to arrange the array:
* 1. Sort the array
* {5, 11, 12, 14, 31}
* 2. Get elements at odd indexes in the array
* to the right
* {5, 12, 31, 11, 14}
* 3. Reverse the sub-array from 0 to (n-1) / 2
* {31, 12, 5, 11, 14}
*/
//Step 1: Sort the array
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int t = arr[j];
arr[j] = arr[j+1];
arr[j+1] = t;
}
}
}
//Step 2: Get elements at odd indexes to the right
int endIdx = n - 1;
int startIdx;
if (n % 2 == 0) {
startIdx = n - 1;
}
else {
startIdx = n - 2;
}
while (startIdx > 0) {
int t = arr[startIdx];
int idx = startIdx;
while (idx != endIdx) {
arr[idx] = arr[idx + 1];
idx++;
}
arr[idx] = t;
startIdx -= 2;
endIdx -= 1;
}
//Step 3: Reverse the sub-array from 0 to (n-1) / 2
for (int i = 0, j = (n - 1) / 2; i < j; i++, j--) {
int t = arr[i];
arr[i] = arr[j];
arr[j] = t;
}
//Print the final array
for (int i = 0; i < n; i++) {
System.out.print(arr[i] + " ");
}
}
}
Output
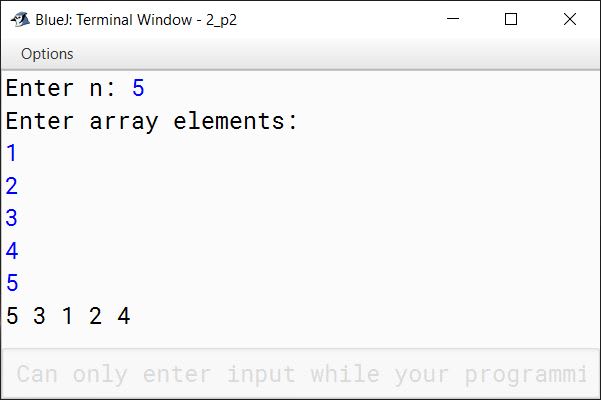
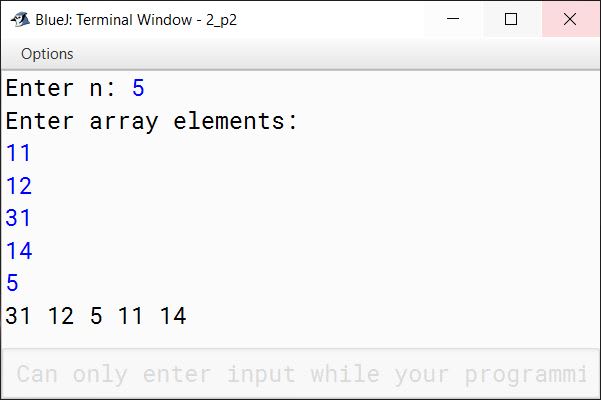
Answered By
5 Likes
Related Questions
The encryption of letters are to be done as follows:
A = 1
B = 2
C = 3 . . .
Z = 26The potential of a word is found by adding the encrypted value of the letters.
Example: KITE
Potential = 11 + 9 + 20 + 5 = 45Accept a sentence which is terminated by either " . " , " ? " or " ! ". Each word of sentence is separated by single space. Decode the words according to their potential and arrange them in ~~alphabetical~~ ~~order~~ increasing order of their potential. Output the result in the format given below:
Example 1
Input:
THE SKY IS THE LIMIT.Potential:
THE = 33
SKY = 55
IS = 28
THE = 33
LIMIT = 63Output:
IS THE THE SKY LIMITExample 2
Input:
LOOK BEFORE YOU LEAP.Potential:
LOOK = 53
BEFORE = 51
YOU = 61
LEAP = 34Output:
LEAP BEFORE LOOK YOUA bank intends to design a program to display the denomination of an input amount, up to 5 digits. The available denomination with the bank are of rupees 2000, 500, 200, 100, 50, 20, 10 and 1.
Design a program to accept the amount from the user and display the break-up in descending order of denominations. (i.e., preference should be given to the highest denomination available) along with the total number of notes.
[Note: Only the denomination used should be displayed].
Also print the amount in words according to the digits.
Example 1:
Input:
14836Output:
One Four Eight Three Six
Denomination:
2000 * 7 = 14000
500 * 1 = 500
200 * 1 = 200
100 * 1 = 100
20 * 1 = 20
10 * 1 = 10
1 * 6 = 6Example 2:
Input:
235001Output:
Invalid AmountWrite a program to input and store n integers (n > 0) in a single subscripted variable and print each number with its frequency. The output should contain number and its frequency in two different columns.
Sample Input:
12 20 14 12 12 20 16 16 14 14 12 20 18 18 Sample Output:
Number Frequency 12 4 14 3 16 2 18 2 20 3 A company manufactures packing cartons in four sizes, i.e. cartons to accommodate 6 boxes, 12 boxes, 24 boxes and 48 boxes. Design a program to accept the number of boxes to be packed (N) by the user (maximum up to 1000 boxes) and display the break-up of the cartons used in descending order of capacity (i.e. preference should be given to the highest capacity available, and if boxes left are less than 6, an extra carton of capacity 6 should be used.)
Test your program with the following data and some random data:
Example 1
INPUT:
N = 726OUTPUT:
48 * 15 = 720
6 * 1 = 6
Remaining boxes = 0
Total number of boxes = 726
Total number of cartons = 16Example 2
INPUT:
N = 140OUTPUT:
48 * 2 = 96
24 * 1 = 24
12 * 1 = 12
6 * 1 = 6
Remaining boxes = 2 * 1 = 2
Total number of boxes = 140
Total number of cartons = 6Example 3
INPUT:
N = 4296OUTPUT:
INVALID INPUT