Computer Science
The encryption of letters are to be done as follows:
A = 1
B = 2
C = 3 . . .
Z = 26
The potential of a word is found by adding the encrypted value of the letters.
Example: KITE
Potential = 11 + 9 + 20 + 5 = 45
Accept a sentence which is terminated by either " . " , " ? " or " ! ". Each word of sentence is separated by single space. Decode the words according to their potential and arrange them in ~~alphabetical~~ ~~order~~ increasing order of their potential. Output the result in the format given below:
Example 1
Input:
THE SKY IS THE LIMIT.
Potential:
THE = 33
SKY = 55
IS = 28
THE = 33
LIMIT = 63
Output:
IS THE THE SKY LIMIT
Example 2
Input:
LOOK BEFORE YOU LEAP.
Potential:
LOOK = 53
BEFORE = 51
YOU = 61
LEAP = 34
Output:
LEAP BEFORE LOOK YOU
Java
Java Arrays
13 Likes
Answer
import java.util.*;
public class KboatWordPotential
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("ENTER THE SENTENCE:");
String ipStr = in.nextLine();
int len = ipStr.length();
char lastChar = ipStr.charAt(len - 1);
if (lastChar != '.'
&& lastChar != '?'
&& lastChar != '!') {
System.out.println("INVALID INPUT");
return;
}
String str = ipStr.substring(0, len - 1);
StringTokenizer st = new StringTokenizer(str);
int wordCount = st.countTokens();
String strArr[] = new String[wordCount];
int potArr[] = new int[wordCount];
for (int i = 0; i < wordCount; i++) {
strArr[i] = st.nextToken();
potArr[i] = computePotential(strArr[i]);
}
System.out.println("Potential:");
for (int i = 0; i < wordCount; i++) {
System.out.println(strArr[i] + " = " + potArr[i]);
}
/*
* Sort potential array and words array
* as per potential array
*/
for (int i = 0; i < wordCount - 1; i++) {
for (int j = 0; j < wordCount - i - 1; j++) {
if (potArr[j] > potArr[j+1]) {
int t = potArr[j];
potArr[j] = potArr[j+1];
potArr[j+1] = t;
String temp = strArr[j];
strArr[j] = strArr[j+1];
strArr[j+1] = temp;
}
}
}
System.out.println("Sorted Sentence");
for (int i = 0; i < wordCount; i++) {
System.out.print(strArr[i] + " ");
}
}
public static int computePotential(String word) {
String str = word.toUpperCase();
int p = 0;
int l = str.length();
/*
* Substracting 64 from ASCII Value of
* letter gives the proper potentials:
* A => 65 - 64 = 1
* B => 66 - 64 = 2
* ..
* ..
*/
for (int i = 0; i < l; i++) {
p += str.charAt(i) - 64;
}
return p;
}
}
Output
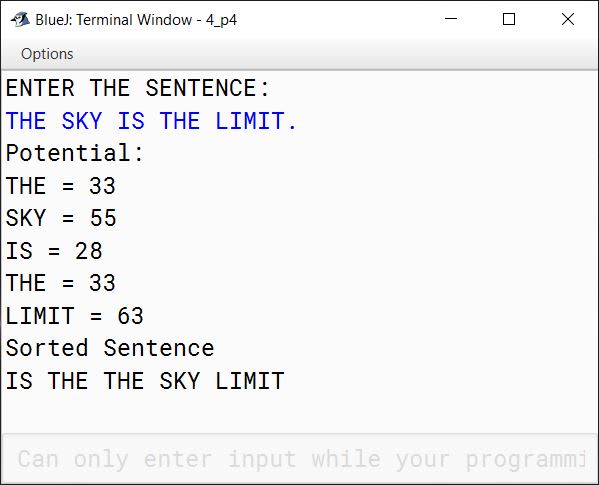
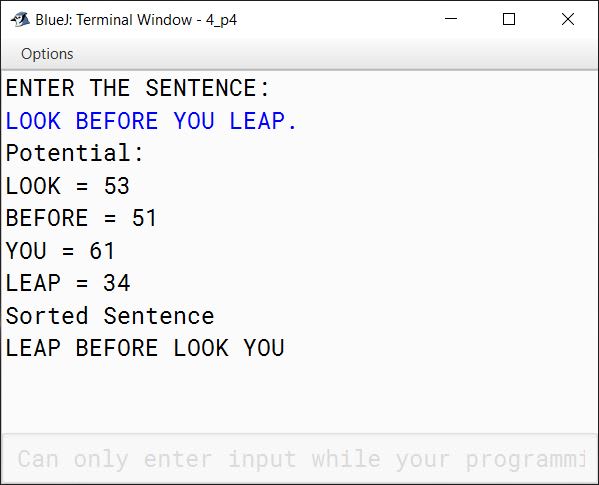
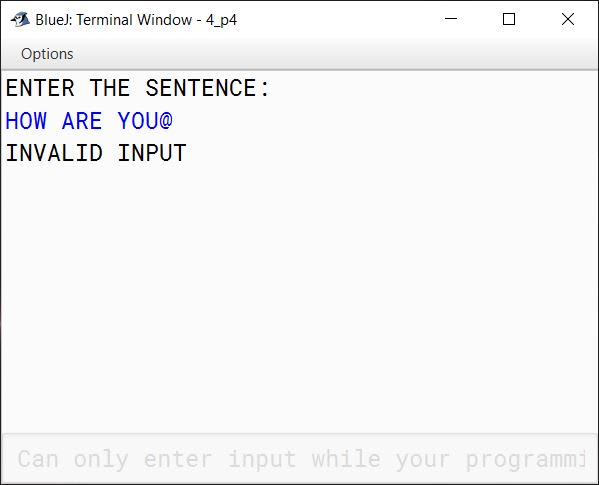
Answered By
3 Likes
Related Questions
A bank intends to design a program to display the denomination of an input amount, up to 5 digits. The available denomination with the bank are of rupees 2000, 500, 200, 100, 50, 20, 10 and 1.
Design a program to accept the amount from the user and display the break-up in descending order of denominations. (i.e., preference should be given to the highest denomination available) along with the total number of notes.
[Note: Only the denomination used should be displayed].
Also print the amount in words according to the digits.
Example 1:
Input:
14836Output:
One Four Eight Three Six
Denomination:
2000 * 7 = 14000
500 * 1 = 500
200 * 1 = 200
100 * 1 = 100
20 * 1 = 20
10 * 1 = 10
1 * 6 = 6Example 2:
Input:
235001Output:
Invalid AmountA company manufactures packing cartons in four sizes, i.e. cartons to accommodate 6 boxes, 12 boxes, 24 boxes and 48 boxes. Design a program to accept the number of boxes to be packed (N) by the user (maximum up to 1000 boxes) and display the break-up of the cartons used in descending order of capacity (i.e. preference should be given to the highest capacity available, and if boxes left are less than 6, an extra carton of capacity 6 should be used.)
Test your program with the following data and some random data:
Example 1
INPUT:
N = 726OUTPUT:
48 * 15 = 720
6 * 1 = 6
Remaining boxes = 0
Total number of boxes = 726
Total number of cartons = 16Example 2
INPUT:
N = 140OUTPUT:
48 * 2 = 96
24 * 1 = 24
12 * 1 = 12
6 * 1 = 6
Remaining boxes = 2 * 1 = 2
Total number of boxes = 140
Total number of cartons = 6Example 3
INPUT:
N = 4296OUTPUT:
INVALID INPUTWrite a program to accept a set of n integers (where n > 0) in a single dimensional array. Arrange the elements of the array such that the lowest number appears in the centre of the array, next lower number in the right cell of the centre, next lower in the left cell of the centre and so on… . The process will stop when the highest number will set in its appropriate cell. Finally, display the array elements.(Pendulum Arrangement of Array)
Assume that the memory space is less. Hence, you don't need to create extra array for the aforesaid task.
Example:
Input: 1 2 3 4 5
Output: 5 3 1 2 4Input: 11 12 31 14 5
Output: 31 12 5 11 14Given a square matrix M[][] of order 'n'. The maximum value possible for 'n' is 10. Accept three different characters from the keyboard and fill the array according to the output shown in the examples. If the value of n exceeds 10 then an appropriate message should be displayed.
Example 1
Enter Size: 4
Input:
First Character '*'
Second Character '?'
Third Character '#'Output:
# * * #
? # # ?
? # # ?
# * * #Example 2
Enter Size: 5
Input:
First Character 'Output:
@ @
! @ @ !
@ $ @Example 3
Enter Size: 65
Output:
Size out of Range