Computer Applications
Write a program in Java, using the Scanner methods, to read and display the following details:
Name - as a String data type
Roll Number - as an integer data type
Marks in 5 subjects - as a float data type
Compute and display the percentage of marks.
Java
Input in Java
34 Likes
Answer
import java.util.Scanner;
public class KboatMarks
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter name: ");
String name = in.nextLine();
System.out.print("Enter Roll Number: ");
int rollNo = in.nextInt();
System.out.print("Enter Marks in 1st Subject: ");
float m1 = in.nextFloat();
System.out.print("Enter Marks in 2nd Subject: ");
float m2 = in.nextFloat();
System.out.print("Enter Marks in 3rd Subject: ");
float m3 = in.nextFloat();
System.out.print("Enter Marks in 4th Subject: ");
float m4 = in.nextFloat();
System.out.print("Enter Marks in 5th Subject: ");
float m5 = in.nextFloat();
float t = m1 + m2 + m3 + m4 + m5;
float p = t / 500 * 100;
System.out.println("Percentage Marks = " + p);
in.close();
}
}
Output
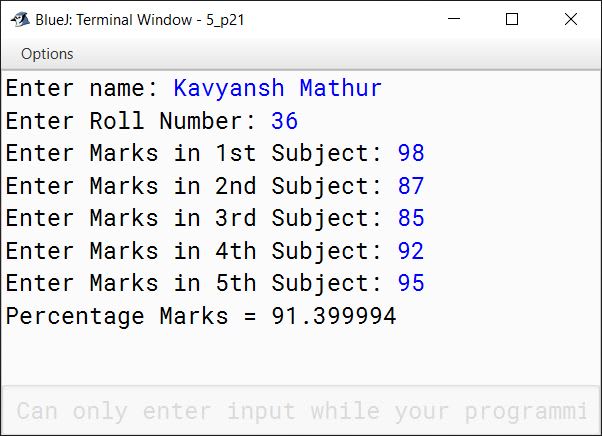
Answered By
8 Likes
Related Questions
Write a program that takes the distance of the commute in kilometres, the car fuel consumption rate in kilometre per gallon, and the price of a gallon of petrol as input. The program should then display the cost of the commute.
Write a Java program that reads a line of text separated by any number of whitespaces and outputs the line with correct spacing, that is, the output has no space before the first word and exactly one space between each pair of adjacent words.
Write a program to compute the Time Period (T) of a Simple Pendulum as per the following formula:
T = 2π√(L/g)
Input the value of L (Length of Pendulum) and g (gravity) using the Scanner class.
Write a program in Java that accepts the seconds as input and converts them into the corresponding number of hours, minutes and seconds. A sample output is shown below:
Enter Total Seconds: 5000 1 Hour(s) 23 Minute(s) 20 Second(s)