Computer Applications
Write a program in Java that accepts the seconds as input and converts them into the corresponding number of hours, minutes and seconds. A sample output is shown below:
Enter Total Seconds:
5000
1 Hour(s) 23 Minute(s) 20 Second(s)
Java
Input in Java
86 Likes
Answer
import java.util.Scanner;
public class KboatTimeConvert
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter time in seconds: ");
long secs = in.nextLong();
long hrs = secs / 3600;
secs %= 3600;
long mins = secs / 60;
secs %= 60;
System.out.println(hrs + " Hour(s) " + mins
+ " Minute(s) " + secs + " Second(s)");
in.close();
}
}
Output
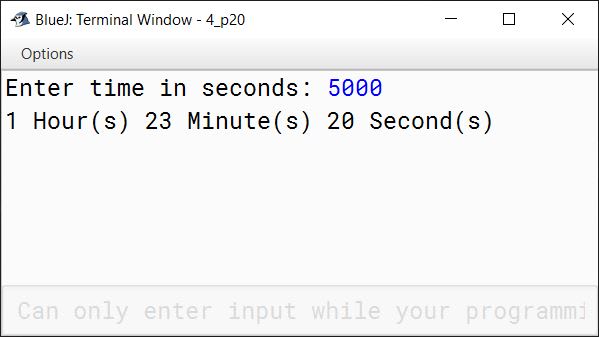
Answered By
33 Likes
Related Questions
Write a Java program that reads a line of text separated by any number of whitespaces and outputs the line with correct spacing, that is, the output has no space before the first word and exactly one space between each pair of adjacent words.
Write a program that takes the distance of the commute in kilometres, the car fuel consumption rate in kilometre per gallon, and the price of a gallon of petrol as input. The program should then display the cost of the commute.
Write a program in Java, using the Scanner methods, to read and display the following details:
Name - as a String data type
Roll Number - as an integer data type
Marks in 5 subjects - as a float data typeCompute and display the percentage of marks.
Write a program to compute the Time Period (T) of a Simple Pendulum as per the following formula:
T = 2π√(L/g)
Input the value of L (Length of Pendulum) and g (gravity) using the Scanner class.