Computer Applications
Write a program in Java to store 10 words in a Single Dimensional Array. Display only those words which are Palindrome.
Sample Input: MADAM, TEACHER, SCHOOL, ABBA, ………
Sample Output: MADAM
ABBA
……….
……….
Java
Java String Handling
53 Likes
Answer
import java.util.Scanner;
public class KboatSDAPalindrome
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
String words[] = new String[10];
System.out.println("Enter 10 words:");
for (int i = 0; i < words.length; i++) {
words[i] = in.nextLine();
}
System.out.println("\nPalindrome Words:");
for (int i = 0; i < words.length; i++) {
String str = words[i].toUpperCase();
int strLen = str.length();
boolean isPalin = true;
for (int j = 0; j < strLen / 2; j++) {
if (str.charAt(j) != str.charAt(strLen - 1 - j)) {
isPalin = false;
break;
}
}
if (isPalin)
System.out.println(words[i]);
}
}
}
Variable Description Table
Program Explanation
Output
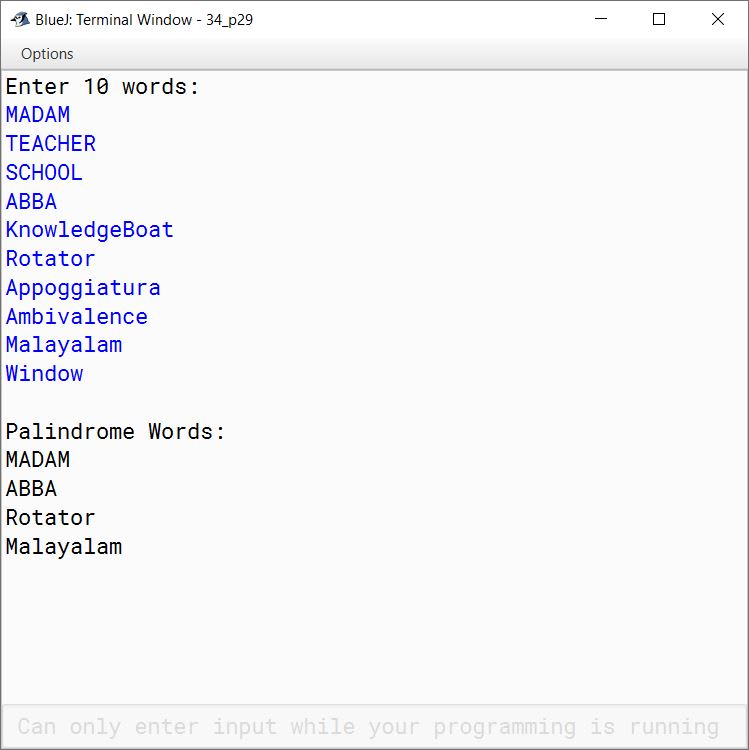
Answered By
14 Likes
Related Questions
Write a program in Java to input the names of 10 cities in a Single Dimensional Array. Display only those names which begin with a consonant but end with a vowel.
Sample Input: Kolkata, Delhi, Bengaluru, Jamshedpur, Bokaro, …….
Sample Output: Kolkata
Delhi
Bengaluru
Bokaro
….
….Write a program to input twenty names in an array. Arrange these names in ascending order of letters, using the bubble sort technique.
Sample Input:
Rohit, Devesh, Indrani, Shivangi, Himanshu, Rishi, Piyush, Deepak, Abhishek, Kunal, …..
Sample Output:
Abhishek, Deepak, Devesh, Himanshu, Indrani, Kunal, Piyush, Rishi, Rohit, Shivangi, …..Write a program to accept 10 names in a Single Dimensional Array (SDA). Display the names whose first letter matches with the letter entered by the user.
Sample Input:
Aman Shahi
Akash Gupta
Suman Mishra
and so on ……….Sample Output:
Enter the alphabet: A
Aman Shahi
Akash Gupta
…..
…..Write a program to accept the names of 10 cities in a single dimensional string array and their STD (Subscribers Trunk Dialling) codes in another single dimension integer array. Search for the name of a city input by the user in the list. If found, display "Search Successful" and print the name of the city along with its STD code, or else display the message "Search unsuccessful, no such city in the list".