Computer Applications
Write a program in Java to read a number, remove all zeros from it, and display the new number. For example,
Sample Input: 45407703
Sample Output: 454773
Java
Java Iterative Stmts
3 Likes
Answer
import java.util.Scanner;
public class KboatRemoveZeros
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number: ");
int num = in.nextInt();
int idx = 0;
int newNum = 0;
int n = num;
while (n != 0) {
int d = n % 10;
if (d != 0) {
newNum += (int)(d * Math.pow(10, idx));
idx++;
}
n /= 10;
}
System.out.println("Original number = " + num);
System.out.println("New number = " + newNum);
}
}
Output
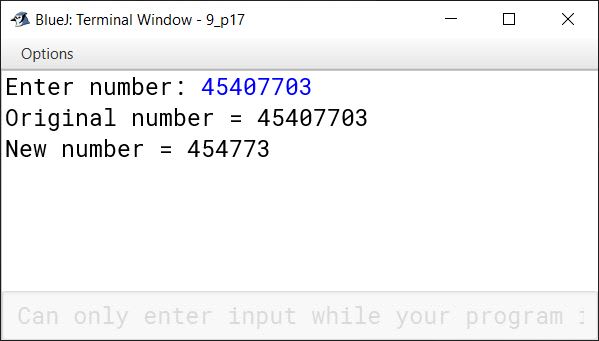
Answered By
2 Likes
Related Questions
Write a program in Java to read a number and display its digits in the reverse order. For example, if the input number is 2468, then the output should be 8642.
Output:
Enter a number: 2468
Original number: 2468
Reverse number: 8642Write a program that displays all the numbers from 150 to 250 that are divisible by 5 or 6, but not both.
Write a program to read the number n via the Scanner class and print the Tribonacci series:
0, 0, 1, 1, 2, 4, 7, 13, 24, 44, 81 …and so on.
Hint: The Tribonacci series is a generalisation of the Fibonacci sequence where each term is the sum of the three preceding terms.
Write a program to calculate the value of Pi with the help of the following series:
Pi = (4/1) - (4/3) + (4/5) - (4/7) + (4/9) - (4/11) + (4/13) - (4/15) …
Hint: Use while loop with 100000 iterations.