Computer Applications
Write a program in Java to read a number and display its digits in the reverse order. For example, if the input number is 2468, then the output should be 8642.
Output:
Enter a number: 2468
Original number: 2468
Reverse number: 8642
Java
Java Iterative Stmts
2 Likes
Answer
import java.util.Scanner;
public class KboatDigitReverse
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Number: ");
int orgNum = in.nextInt();
int copyNum = orgNum;
int revNum = 0;
while(copyNum != 0) {
int digit = copyNum % 10;
copyNum /= 10;
revNum = revNum * 10 + digit;
}
System.out.println("Original Number = " + orgNum);
System.out.println("Reverse Number = " + revNum);
}
}
Output
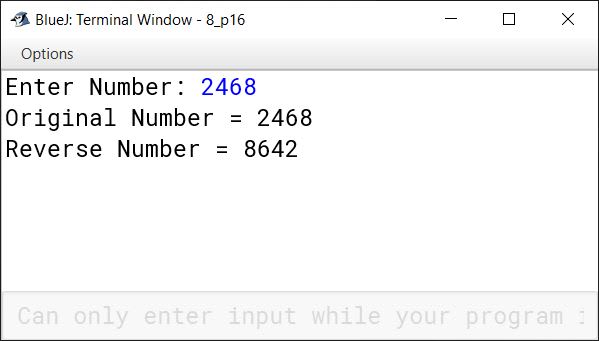
Answered By
2 Likes
Related Questions
Write a program to convert kilograms to pounds in the following tabular format (1 kilogram is 2.2 pounds):
Kilograms Pounds 1 2.2 2 4.4 20 44.0 Write a program to read the number n via the Scanner class and print the Tribonacci series:
0, 0, 1, 1, 2, 4, 7, 13, 24, 44, 81 …and so on.
Hint: The Tribonacci series is a generalisation of the Fibonacci sequence where each term is the sum of the three preceding terms.
Write a program that displays all the numbers from 150 to 250 that are divisible by 5 or 6, but not both.
Write a program in Java to read a number, remove all zeros from it, and display the new number. For example,
Sample Input: 45407703
Sample Output: 454773