Computer Applications
Write a program in Java to input two numbers (say, n and m) using the Scanner class. Display the results of n raised to the power m (nm) and mn.
Java
Input in Java
26 Likes
Answer
import java.util.*;
public class KboatExponent
{
public static void main(String args[])
{
int n, m;
double expo;
Scanner in = new Scanner(System.in);
System.out.print("Enter a number (n): ");
n = in.nextInt();
System.out.print("Enter a number (m): ");
m = in.nextInt();
expo = Math.pow(n,m);
System.out.println(n + " raised to the power "
+ m + " = " + expo);
expo = Math.pow(m,n);
System.out.println(m + " raised to the power "
+ n + " = " + expo);
}
}
Output
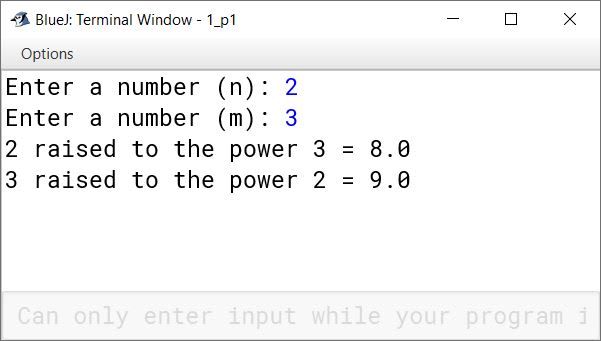
Answered By
16 Likes
Related Questions
Write a program in Java to input perpendicular and base of a right angle triangle using the Scanner class. Calculate and display its hypotenuse using the formula given below:
h = √(p2 + b2)A triangle is said to be an 'Equable Triangle', if the area of the triangle is equal to its perimeter. Write a program to enter three sides of a triangle. Check and print whether the triangle is equable or not.
For example, a right angled triangle with sides 5, 12 and 13 has its area and perimeter both equal to 30.A shopkeeper offers 30% discount on purchasing articles whereas the other shopkeeper offers two successive discounts 20% and 10% for purchasing the same articles. Write a program in Java to compute and display the discounts.
Take the price of an article as the input.Write a program to input the cost price and the selling price of an article. If the selling price is more than the cost price then calculate and display actual profit and profit per cent otherwise, calculate and display actual loss and loss per cent. If the cost price and the selling price are equal, the program displays the message 'Neither profit nor loss'.