Computer Applications
Write a program in Java to input a series of numbers one by one to print the count and average of those numbers which have 3 as their last digit. The process of inputting numbers should stop if the number inputted by the user is a negative number.
Java
Java Iterative Stmts
1 Like
Answer
import java.util.Scanner;
public class KboatNumbers
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter the numbers: ");
int count = 0;
double sum = 0;
while (true) {
int n = in.nextInt();
if (n < 0) {
break;
}
int ld = n % 10;
if (ld == 3) {
count++;
sum += n;
}
}
double avg = sum / count;
System.out.println("Count = " + count);
System.out.println("Average = " + avg);
}
}
Output
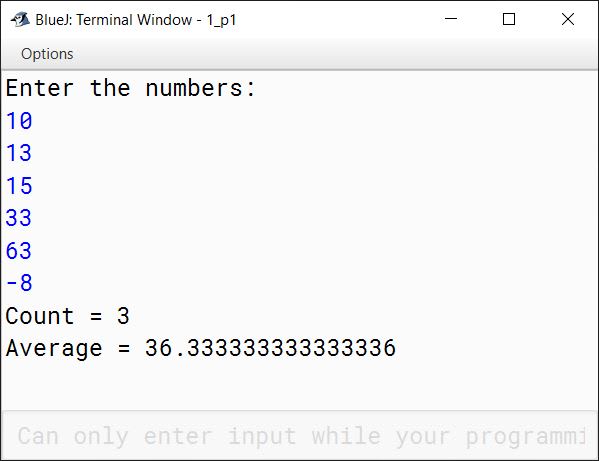
Answered By
3 Likes
Related Questions
Define a class to accept a number and check whether it is a SUPERSPY number or not. A number is called SUPERSPY if the sum of the digits equals the number of the digits.
Example1:
Input: 1021 output: SUPERSPY number [SUM OF THE DIGITS = 1+0+2+1 = 4, NUMBER OF DIGITS = 4 ]
Example2:
Input: 125 output: Not an SUPERSPY number [1+2+5 is not equal to 3]
To execute a loop 5 times, which of the following is correct?
Write a program to input a number and find whether the number is an emirp number or not. A number is said to be emirp if the original number and the reversed number both are prime numbers.
For example, 17 is an emirp number as 17 and its reverse 71 are both prime numbers.
Define a class to accept a number from user and check if it is an EvenPal number or not.
(The number is said to be EvenPal number when number is palindrome number (a number is palindrome if it is equal to its reverse) and sum of its digits is an even number.)
Example: 121 – is a palindrome number
Sum of the digits – 1+2+1 = 4 which is an even number