Computer Applications
Write a program in Java to accept the name of an employee and his/her annual income. Pass the name and the annual income to a method Tax(String name, int income) which displays the name of the employee and the income tax as per the given tariff:
Annual Income | Income Tax |
---|---|
Up to ₹2,50,000 | No tax |
₹2,50,001 to ₹5,00,000 | 10% of the income exceeding ₹2,50,000 |
₹5,00,001 to ₹10,00,000 | ₹30,000 + 20% of the amount exceeding ₹5,00,000 |
₹10,00,001 and above | ₹50,000 + 30% of the amount exceeding ₹10,00,000 |
Java
User Defined Methods
72 Likes
Answer
import java.util.Scanner;
public class KboatEmployeeTax
{
public void tax(String name, int income) {
double tax;
if (income <= 250000)
tax = 0;
else if (income <= 500000)
tax = (income - 250000) * 0.1;
else if (income <= 1000000)
tax = 30000 + ((income - 500000) * 0.2);
else
tax = 50000 + ((income - 1000000) * 0.3);
System.out.println("Name: " + name);
System.out.println("Income Tax: " + tax);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter name: ");
String n = in.nextLine();
System.out.print("Enter annual income: ");
int i = in.nextInt();
KboatEmployeeTax obj = new KboatEmployeeTax();
obj.tax(n, i);
}
}
Variable Description Table
Program Explanation
Output
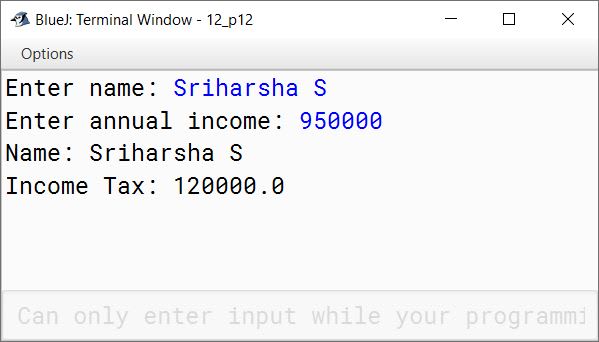
Answered By
26 Likes
Related Questions
Write a program in Java to accept a String from the user. Pass the String to a method Change(String str) which displays the first character of each word after changing the case (lower to upper and vice versa).
Sample Input: Delhi public school
Sample Output:
d
P
SWrite a program to accept 10 numbers in a Single Dimensional Array. Pass the array to a method Search(int m[], int ns) to search the given number ns in the list of array elements. If the number is present, then display the message 'Number is present' otherwise, display 'number is not present'.
Write a program in Java to accept a String from the user. Pass the String to a method Display(String str) which displays the consonants present in the String.
Sample Input: computer
Sample Output:
c
m
p
t
rWrite a program in Java to accept a String from the user. Pass the String to a method First(String str) which displays the first character of each word.
Sample Input : Understanding Computer Applications
Sample Output:
U
C
A