Computer Applications
A university student's registration number follows the format:
<CourseCode><Year><CollegeCode><RollNumber>
where
Component | Description |
---|---|
CourseCode | A 3-letter code representing the course (e.g., CSE for Computer Science, ECE for Electronics & Communication) |
Year | The last two digits of the admission year. |
CollegeCode | A 3-digit code representing the college |
RollNumber | A 4-digit unique student roll number. |
Examples
Registration Number | Course Code | Admission Year | College Code | Roll Number |
---|---|---|---|---|
CSE240011023 | CSE | 24 | 001 | 1023 |
ECE252104297 | ECE | 25 | 210 | 4297 |
ASE230277259 | ASE | 23 | 027 | 7259 |
Define a class that accepts a student's registration number as input, extracts the relevant details, and displays them in the specified format.
import java.util.Scanner;
public class KboatStuRegNum
{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter reg. no.: ");
_______(1)_________
int l = n.length();
if (l != 12) {
System.out.println("Invalid reg. no.");
System.exit(0);
}
_______(2)_________
_______(3)_________
_______(4)_________
_______(5)_________
System.out.println("Course Code : " + cc);
System.out.println("Admission Year : " + yr);
System.out.println("College Code : " + cl);
System.out.println("Roll Number : " + rNo);
}
}
Java String Handling
3 Likes
Answer
String n = in.nextLine();
String cc = n.substring(0, 3);
String yr = n.substring(3, 5);
String cl = n.substring(5, 8);
String rNo = n.substring(8);
Explanation
import java.util.Scanner;
public class KboatStuRegNum
{
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("Enter reg. no.: ");
String n = in.nextLine();
int l = n.length();
if (l != 12) {
System.out.println("Invalid reg. no.");
System.exit(0);
}
String cc = n.substring(0, 3);
String yr = n.substring(3, 5);
String cl = n.substring(5, 8);
String rNo = n.substring(8);
System.out.println("Course Code : " + cc);
System.out.println("Admission Year : " + yr);
System.out.println("College Code : " + cl);
System.out.println("Roll Number : " + rNo);
}
}
Variable Description Table
Variable Name | Data Type | Purpose |
---|---|---|
in | Scanner | An instance of the Scanner class used to read input from the user. |
n | String | Stores the registration number entered by the user. |
l | int | Stores the length of the registration number entered by the user. |
cc | String | Extracts the course code from the registration number (first 3 characters). |
yr | String | Extracts the admission year from the registration number (4th and 5th characters). |
cl | String | Extracts the college code from the registration number (6th to 8th characters). |
rNo | String | Extracts the roll number from the registration number (last 4 characters). |
Program Explanation
Let’s take a closer look at this Java program to understand how it works:
1. Importing Scanner:
- The program starts by importing the
java.util.Scanner
class, which is used for reading input from the user. TheScanner
class is a part of Java's standard library that allows us to capture input from various input sources, including the keyboard.
2. Defining the Main Class & main
Method:
- The class is named
KboatStuRegNum
. It contains themain
method which is the entry point of the program. It is where the program begins execution.
3. Reading Input:
- A
Scanner
object namedin
is created to capture user input. The program then prompts the user to "Enter reg. no." withSystem.out.print
.
4. Getting the Registration Number:
- Using
in.nextLine()
, it reads a line of text input from the user and stores it in theString
variablen
.
5. Validation:
- The program checks if the length of the registration number (
n.length()
) is exactly 12 characters long. If the length isn't 12, it displays "Invalid reg. no." and terminates the program usingSystem.exit(0)
. This ensures the input adheres to the expected format.
6. Extracting Information:
- Assuming the input is valid (i.e., 12 characters), the program extracts different parts of the registration number using the
substring
method:cc
: Extracts the first three characters (n.substring(0, 3)
) representing the course code.yr
: Extracts the next two characters (n.substring(3, 5)
) representing the admission year.cl
: Extracts characters six to eight (n.substring(5, 8)
) which are expected to represent the college code.rNo
: Extracts the remaining characters from the ninth to twelfth position (n.substring(8)
) which are considered the roll number.
7. Displaying the Components:
- The program then outputs the separated components of the registration number to the console using
System.out.println()
. This includes:- "Course Code : " followed by the extracted course code.
- "Admission Year : " followed by the extracted admission year.
- "College Code : " followed by the extracted college code.
- "Roll Number : " followed by the extracted roll number.
Output
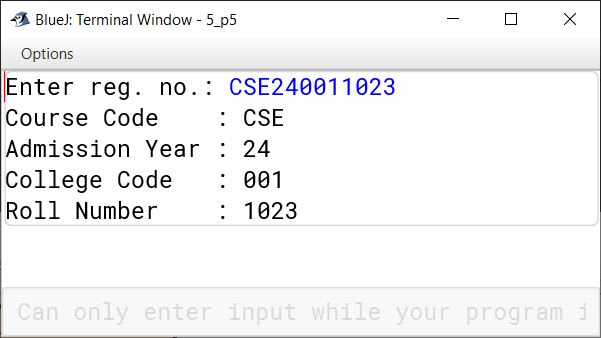
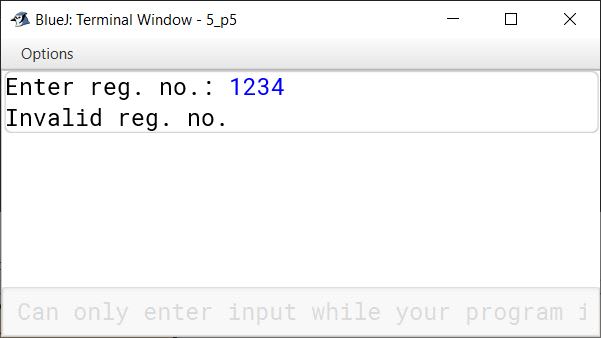
Answered By
3 Likes
Related Questions
A shopping website offers a special discount if the order ID has the sequence 555 anywhere in it. For example, 158545553031, 198555267140, …. .
Fill in the blanks (a) and (b) in the given Java Method to convert the order ID (a long integer) into a string and check if the sequence 555 is present in it.
void checkOrder(long oid) { String str = _______(a)_________; if(______(b)_______) { System.out.println("Special Discount Eligible: " + oid); } }
Which of the following returns a String?
- length()
- charAt(int)
- replace(char, char)
- indexOf(String)
Write a Java program to enter any sentence and convert the sentence to uppercase. Print only those words of the sentence whose first and last letters are the same.
Two strings,
city1
andcity2
, are compared usingcity1.compareTo(city2)
, and the result is less than zero. What does this indicate?