Computer Applications
The volume of solids, viz. cuboid, cylinder and cone can be calculated by the formula:
- Volume of a cuboid (v = l*b*h)
- Volume of a cylinder (v = π*r2*h)
- Volume of a cone (v = (1/3)*π*r2*h)
Using a switch case statement, write a program to find the volume of different solids by taking suitable variables and data types.
Java
Java Conditional Stmts
146 Likes
Answer
import java.util.Scanner;
public class KboatMenuVolume
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Volume of cuboid");
System.out.println("2. Volume of cylinder");
System.out.println("3. Volume of cone");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
switch(choice) {
case 1:
System.out.print("Enter length of cuboid: ");
double l = in.nextDouble();
System.out.print("Enter breadth of cuboid: ");
double b = in.nextDouble();
System.out.print("Enter height of cuboid: ");
double h = in.nextDouble();
double vol = l * b * h;
System.out.println("Volume of cuboid = " + vol);
break;
case 2:
System.out.print("Enter radius of cylinder: ");
double rCylinder = in.nextDouble();
System.out.print("Enter height of cylinder: ");
double hCylinder = in.nextDouble();
double vCylinder = (22 / 7.0) * Math.pow(rCylinder, 2) * hCylinder;
System.out.println("Volume of cylinder = " + vCylinder);
break;
case 3:
System.out.print("Enter radius of cone: ");
double rCone = in.nextDouble();
System.out.print("Enter height of cone: ");
double hCone = in.nextDouble();
double vCone = (1 / 3.0) * (22 / 7.0) * Math.pow(rCone, 2) * hCone;
System.out.println("Volume of cone = " + vCone);
break;
default:
System.out.println("Wrong choice! Please select from 1 or 2 or 3.");
}
}
}
Variable Description Table
Program Explanation
Output
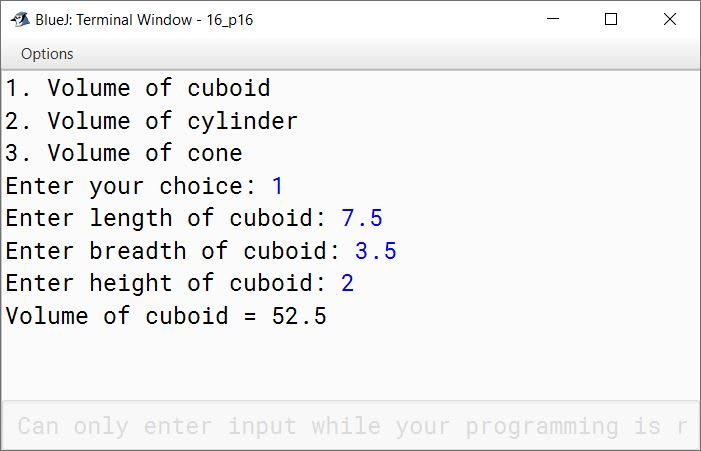
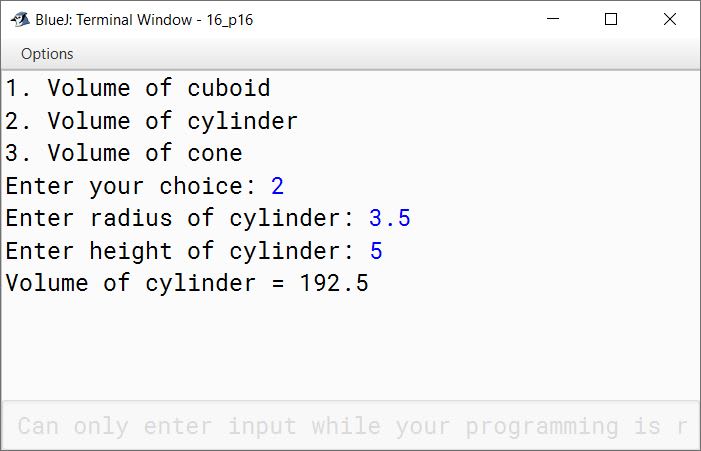
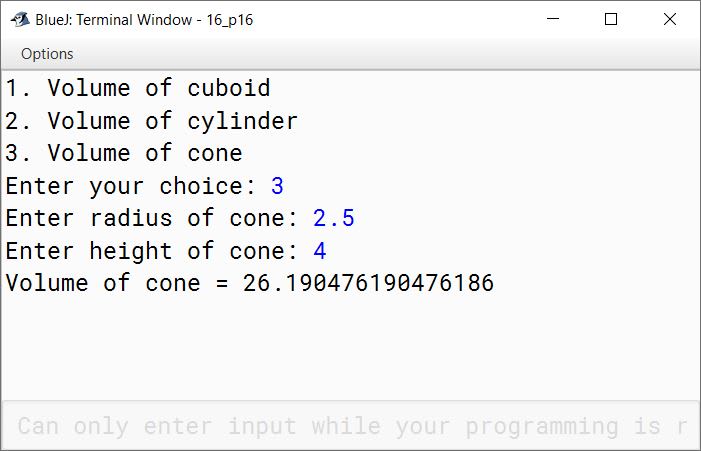
Answered By
46 Likes
Related Questions
A Mega Shop has different floors which display varieties of dresses as mentioned
below:- Ground floor : Kids Wear
- First floor : Ladies Wear
- Second floor : Designer Sarees
- Third Floor : Men's Wear
The user enters floor number and gets the information regarding different items of the Mega shop. After shopping, the customer pays the amount at the billing counter and the shopkeeper prints the bill in the given format:
Name of the Shop: City Mart
Total Amount:
Visit Again!!Write a program to perform the above task as per the user's choice.
The equivalent resistance of series and parallel connections of two resistances are given by the formula:
(a) R1 = r1 + r2 (Series)
(b) R2 = (r1 * r2) / (r1 + r2) (Parallel)
Using a switch case statement, write a program to enter the value of r1 and r2. Calculate and display the equivalent resistances accordingly.
A company announces revised Dearness Allowance (DA) and Special Allowances (SA) for their employees as per the tariff given below:
Basic Dearness Allowance (DA) Special Allowance (SA) Up to ₹ 10,000 10% 5% ₹ 10,001 - ₹ 20,000 12% 8% ₹ 20,001 - ₹ 30,000 15% 10% ₹ 30,001 and above 20% 12% Write a program to accept name and Basic Salary (BS) of an employee. Calculate and display gross salary.
Gross Salary = Basic + Dearness Allowance + Special Allowance
Print the information in the given format:
Name Basic DA Spl. Allowance Gross Salary
xxx xxx xxx xxx xxxUsing a switch case statement, write a menu driven program to convert a given temperature from Fahrenheit to Celsius and vice-versa. For an incorrect choice, an appropriate message should be displayed.
Hint: c = 5/9(f-32) and f=1.8c+32