Computer Applications
The equivalent resistance of series and parallel connections of two resistances are given by the formula:
(a) R1 = r1 + r2 (Series)
(b) R2 = (r1 * r2) / (r1 + r2) (Parallel)
Using a switch case statement, write a program to enter the value of r1 and r2. Calculate and display the equivalent resistances accordingly.
Java
Java Conditional Stmts
117 Likes
Answer
import java.util.Scanner;
public class KboatResistance
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Series");
System.out.println("2. Parallel");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
boolean isChoiceValid = true;
System.out.print("Enter r1: ");
double r1 = in.nextDouble();
System.out.print("Enter r2: ");
double r2 = in.nextDouble();
double eqr = 0.0;
switch (choice) {
case 1:
eqr = r1 + r2;
break;
case 2:
eqr = (r1 * r2) / (r1 + r2);
break;
default:
isChoiceValid = false;
System.out.println("Incorrect choice");
break;
}
if (isChoiceValid)
System.out.println("Equivalent resistance = " + eqr);
}
}
Variable Description Table
Program Explanation
Output
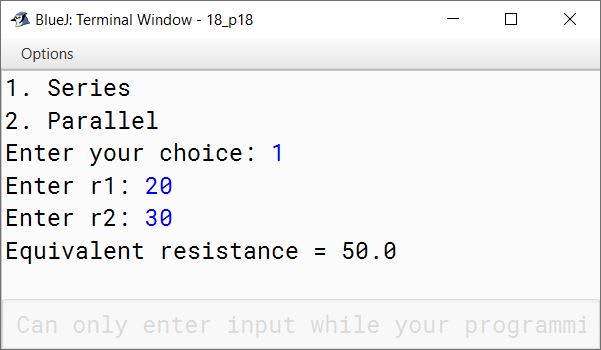
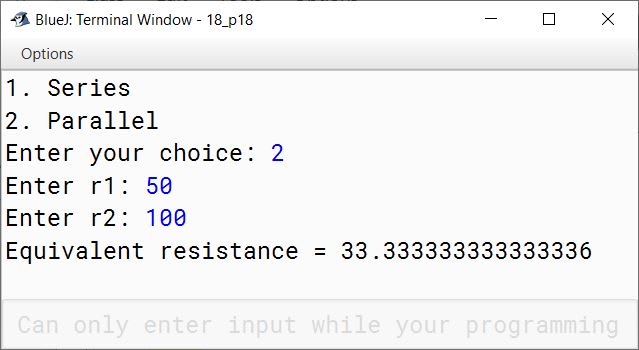
Answered By
39 Likes
Related Questions
The volume of solids, viz. cuboid, cylinder and cone can be calculated by the formula:
- Volume of a cuboid (v = l*b*h)
- Volume of a cylinder (v = π*r2*h)
- Volume of a cone (v = (1/3)*π*r2*h)
Using a switch case statement, write a program to find the volume of different solids by taking suitable variables and data types.
A Mega Shop has different floors which display varieties of dresses as mentioned
below:- Ground floor : Kids Wear
- First floor : Ladies Wear
- Second floor : Designer Sarees
- Third Floor : Men's Wear
The user enters floor number and gets the information regarding different items of the Mega shop. After shopping, the customer pays the amount at the billing counter and the shopkeeper prints the bill in the given format:
Name of the Shop: City Mart
Total Amount:
Visit Again!!Write a program to perform the above task as per the user's choice.
The Simple Interest (SI) and Compound Interest (CI) of a sum (P) for a given time (T) and rate (R) can be calculated as:
(a) SI = (p * r * t) / 100
(b) CI = P * ((1 + (R / 100))T - 1)
Write a program to input sum, rate, time and type of Interest ('S' for Simple Interest and 'C' for Compound Interest). Calculate and display the sum and the interest earned.
'Kumar Electronics' has announced the following seasonal discounts on purchase of certain items.
Purchase Amount Discount on Laptop Discount on Desktop PC Up to ₹ 25000 0.0% 5.0% ₹ 25,001 to ₹ 50,000 5% 7.5% ₹ 50,001 to ₹ 1,00,000 7.5% 10.0% More than ₹ 1,00,000 10.0% 15.0% Write a program to input name, amount of purchase and the type of purchase (`L' for Laptop and 'D' for Desktop) by a customer. Compute and print the net amount to be paid by a customer along with his name.
(Net amount = Amount of purchase - discount)