Computer Applications
The electricity board charges the bill according to the number of units consumed and the rate as given below:
Units Consumed | Rate Per Unit |
---|---|
First 100 units | 80 Paisa per unit |
Next 200 units | Rs. 1 per unit |
Above 300 units | Rs. 2.50 per unit |
Write a program in Java to accept the total units consumed by a customer and calculate the bill. Assume that a meter rent of Rs. 500 is charged from the customer.
Java
Java Conditional Stmts
106 Likes
Answer
import java.util.Scanner;
public class KboatElectricBill
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Units Consumed: ");
int units = in.nextInt();
double amt = 0.0;
if (units <= 100)
amt = units * 0.8;
else if (units <= 300)
amt = (100 * 0.8) + ((units - 100) * 1);
else
amt = (100 * 0.8) + (200 * 1.0) + ((units - 300) * 2.5);
amt += 500;
System.out.println("Units Consumed: " + units);
System.out.println("Bill Amount: " + amt);
}
}
Output
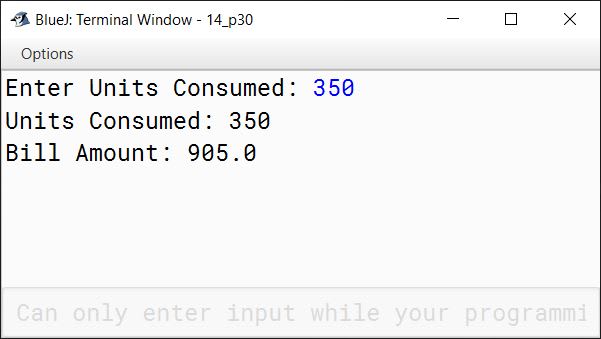
Answered By
47 Likes
Related Questions
Mayur Transport Company charges for parcels as per the following tariff:
Weight Charges Upto 10 Kg. Rs. 30 per Kg. For the next 20 Kg. Rs. 20 per Kg. Above 30 Kg. Rs. 15 per Kg. Write a program in Java to calculate the charge for a parcel, taking the weight of the parcel as an input.
Create a program in Java to find out if a number entered by the user is a Duck Number.
A Duck Number is a number which has zeroes present in it, but there should be no zero present in the beginning of the number. For example, 6710, 8066, 5660303 are all duck numbers whereas 05257, 080009 are not.
Write a menu driven program to accept a number from the user and check whether it is a Buzz number or an Automorphic number.
i. Automorphic number is a number, whose square's last digit(s) are equal to that number. For example, 25 is an automorphic number, as its square is 625 and 25 is present as the last two digits.
ii. Buzz number is a number, that ends with 7 or is divisible by 7.Write a Java program in which you input students name, class, roll number, and marks in 5 subjects. Find out the total marks, percentage, and grade according to the following table.
Percentage Grade >= 90 A+ >= 80 and < 90 A >= 70 and < 80 B+ >= 60 and < 70 B >= 50 and < 60 C >= 40 and < 50 D < 40 E