Computer Applications
The basic salary of employees is undergoing a revision. Define a class called Grade_Revision with the following specifications:
Data Members | Purpose |
---|---|
String name | to store name of the employee |
int bas | to store basic salary |
int expn | to consider the length of service as an experience |
double inc | to store increment |
double nbas | to store new basic salary (basic + increment) |
Member Methods | Purpose |
---|---|
Grade_Revision() | constructor to initialize all data members |
void accept() | to input name, basic and experience |
void increment() | to calculate increment based on experience as per the table given below |
void display() | to print all the details of an employee |
Experience | Increment |
---|---|
Up to 3 years | ₹1,000 + 10% of basic |
3 years or more and up to 5 years | ₹3,000 + 12% of basic |
5 years or more and up to 10 years | ₹5,000 + 15% of basic |
10 years or more | ₹8,000 + 20% of basic |
Write the main method to create an object of the class and call all the member methods.
Java
Java Constructors
61 Likes
Answer
import java.util.Scanner;
public class Grade_Revision
{
private String name;
private int bas;
private int expn;
private double inc;
private double nbas;
public Grade_Revision() {
name = "";
bas = 0;
expn = 0;
inc = 0.0;
nbas = 0.0;
}
public void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter name: ");
name = in.nextLine();
System.out.print("Enter basic: ");
bas = in.nextInt();
System.out.print("Enter experience: ");
expn = in.nextInt();
}
public void increment() {
if (expn <= 3)
inc = 1000 + (bas * 0.1);
else if (expn <= 5)
inc = 3000 + (bas * 0.12);
else if (expn <= 10)
inc = 5000 + (bas * 0.15);
else
inc = 8000 + (bas * 0.2);
nbas = bas + inc;
}
public void display() {
System.out.println("Name: " + name);
System.out.println("Basic: " + bas);
System.out.println("Experience: " + expn);
System.out.println("Increment: " + inc);
System.out.println("New Basic: " + nbas);
}
public static void main(String args[]) {
Grade_Revision obj = new Grade_Revision();
obj.accept();
obj.increment();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
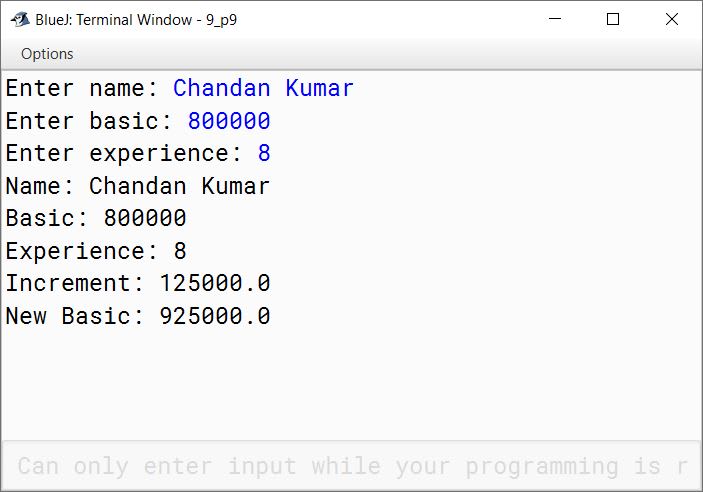
Answered By
23 Likes
Related Questions
Write a program in Java to find the roots of a quadratic equation ax2+bx+c=0 with the following specifications:
Class name — Quad
Data Members — float a,b,c,d (a,b,c are the co-efficients & d is the discriminant), r1 and r2 are the roots of the equation.
Member Methods:
- quad(int x,int y,int z) — to initialize a=x, b=y, c=z, d=0
- void calculate() — Find d=b2-4ac
If d < 0 then print "Roots not possible" otherwise find and print:
r1 = (-b + √d) / 2a
r2 = (-b - √d) / 2aDefine a class named FruitJuice with the following description:
Data Members Purpose int product_code stores the product code number String flavour stores the flavour of the juice (e.g., orange, apple, etc.) String pack_type stores the type of packaging (e.g., tera-pack, PET bottle, etc.) int pack_size stores package size (e.g., 200 mL, 400 mL, etc.) int product_price stores the price of the product Member Methods Purpose FruitJuice() constructor to initialize integer data members to 0 and string data members to "" void input() to input and store the product code, flavour, pack type, pack size and product price void discount() to reduce the product price by 10 void display() to display the product code, flavour, pack type, pack size and product price Define a class called Student to check whether a student is eligible for taking admission in class XI with the following specifications:
Data Members Purpose String name to store name int mm to store marks secured in Maths int scm to store marks secured in Science double comp to store marks secured in Computer Member Methods Purpose Student( ) parameterised constructor to initialize the data members by accepting the details of a student check( ) to check the eligibility for course based on the table given below void display() to print the eligibility by using check() function in nested form Marks Eligibility 90% or more in all the subjects Science with Computer Average marks 90% or more Bio-Science Average marks 80% or more and less than 90% Science with Hindi Write the main method to create an object of the class and call all the member methods.
Define a class Bill that calculates the telephone bill of a consumer with the following description:
Data Members Purpose int bno bill number String name name of consumer int call no. of calls consumed in a month double amt bill amount to be paid by the person Member Methods Purpose Bill() constructor to initialize data members with default initial value Bill(…) parameterised constructor to accept billno, name and no. of calls consumed Calculate() to calculate the monthly telephone bill for a consumer as per the table given below Display() to display the details Units consumed Rate First 100 calls ₹0.60 / call Next 100 calls ₹0.80 / call Next 100 calls ₹1.20 / call Above 300 calls ₹1.50 / call Fixed monthly rental applicable to all consumers: ₹125
Create an object in the main() method and invoke the above functions to perform the desired task.