Computer Applications
Define a class called Student to check whether a student is eligible for taking admission in class XI with the following specifications:
Data Members | Purpose |
---|---|
String name | to store name |
int mm | to store marks secured in Maths |
int scm | to store marks secured in Science |
double comp | to store marks secured in Computer |
Member Methods | Purpose |
---|---|
Student( ) | parameterised constructor to initialize the data members by accepting the details of a student |
check( ) | to check the eligibility for course based on the table given below |
void display() | to print the eligibility by using check() function in nested form |
Marks | Eligibility |
---|---|
90% or more in all the subjects | Science with Computer |
Average marks 90% or more | Bio-Science |
Average marks 80% or more and less than 90% | Science with Hindi |
Write the main method to create an object of the class and call all the member methods.
Java
Java Constructors
54 Likes
Answer
import java.util.Scanner;
public class Student
{
private String name;
private int mm;
private int scm;
private int comp;
public Student(String n, int m, int sc, int c) {
name = n;
mm = m;
scm = sc;
comp = c;
}
private String check() {
String course = "Not Eligible";
double avg = (mm + scm + comp) / 3.0;
if (mm >= 90 && scm >= 90 && comp >= 90)
course = "Science with Computer";
else if (avg >= 90)
course = "Bio-Science";
else if (avg >= 80)
course = "Science with Hindi";
return course;
}
public void display() {
String eligibility = check();
System.out.println("Eligibility: " + eligibility);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String n = in.nextLine();
System.out.print("Enter Marks in Maths: ");
int maths = in.nextInt();
System.out.print("Enter Marks in Science: ");
int sci = in.nextInt();
System.out.print("Enter Marks in Computer: ");
int compSc = in.nextInt();
Student obj = new Student(n, maths, sci, compSc);
obj.display();
}
}
Variable Description Table
Program Explanation
Output
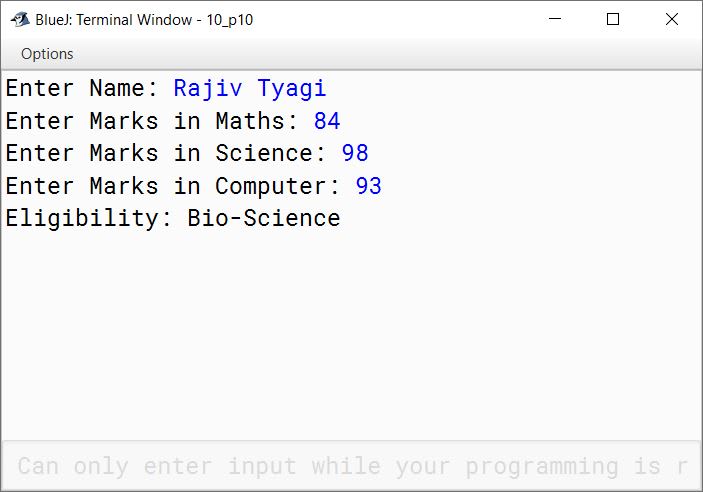
Answered By
20 Likes
Related Questions
Define a class Bill that calculates the telephone bill of a consumer with the following description:
Data Members Purpose int bno bill number String name name of consumer int call no. of calls consumed in a month double amt bill amount to be paid by the person Member Methods Purpose Bill() constructor to initialize data members with default initial value Bill(…) parameterised constructor to accept billno, name and no. of calls consumed Calculate() to calculate the monthly telephone bill for a consumer as per the table given below Display() to display the details Units consumed Rate First 100 calls ₹0.60 / call Next 100 calls ₹0.80 / call Next 100 calls ₹1.20 / call Above 300 calls ₹1.50 / call Fixed monthly rental applicable to all consumers: ₹125
Create an object in the main() method and invoke the above functions to perform the desired task.
Define a class called BookFair with the following description:
Data Members Purpose String Bname stores the name of the book double price stores the price of the book Member Methods Purpose BookFair( ) Constructor to initialize data members void input( ) To input and store the name and price of the book void calculate( ) To calculate the price after discount. Discount is calculated as per the table given below void display( ) To display the name and price of the book after discount Price Discount Less than or equal to ₹1000 2% of price More than ₹1000 and less than or equal to ₹3000 10% of price More than ₹3000 15% of price Write a main method to create an object of the class and call the above member methods.
Define a class named FruitJuice with the following description:
Data Members Purpose int product_code stores the product code number String flavour stores the flavour of the juice (e.g., orange, apple, etc.) String pack_type stores the type of packaging (e.g., tera-pack, PET bottle, etc.) int pack_size stores package size (e.g., 200 mL, 400 mL, etc.) int product_price stores the price of the product Member Methods Purpose FruitJuice() constructor to initialize integer data members to 0 and string data members to "" void input() to input and store the product code, flavour, pack type, pack size and product price void discount() to reduce the product price by 10 void display() to display the product code, flavour, pack type, pack size and product price The basic salary of employees is undergoing a revision. Define a class called Grade_Revision with the following specifications:
Data Members Purpose String name to store name of the employee int bas to store basic salary int expn to consider the length of service as an experience double inc to store increment double nbas to store new basic salary (basic + increment) Member Methods Purpose Grade_Revision() constructor to initialize all data members void accept() to input name, basic and experience void increment() to calculate increment based on experience as per the table given below void display() to print all the details of an employee Experience Increment Up to 3 years ₹1,000 + 10% of basic 3 years or more and up to 5 years ₹3,000 + 12% of basic 5 years or more and up to 10 years ₹5,000 + 15% of basic 10 years or more ₹8,000 + 20% of basic Write the main method to create an object of the class and call all the member methods.