Computer Applications
Mr. Kumar is an LIC agent. He offers discount to his policy holders on the annual premium. However, he also gets commission on the sum assured as per the given tariff.
Sum Assured | Discount | Commission |
---|---|---|
Up to ₹ 1,00,000 | 5% | 2% |
₹ 1,00,001 and up to ₹ 2,00,000 | 8% | 3% |
₹ 2,00,001 and up to ₹ 5,00,000 | 10% | 5% |
More than ₹ 5,00,000 | 15% | 7.5% |
Write a program to input name of the policy holder, the sum assured and first annual premium. Calculate the discount of the policy holder and the commission of the agent. The program displays all the details as:
Name of the policy holder :
Sum assured :
Premium :
Discount on the first premium :
Commission of the agent :
Java
Java Conditional Stmts
148 Likes
Answer
import java.util.Scanner;
public class KboatLICPolicy
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String name = in.nextLine();
System.out.print("Enter Sum Assured: ");
double sum = in.nextDouble();
System.out.print("Enter First Premium: ");
double pre = in.nextDouble();
double disc = 0.0, comm = 0.0;
if(sum <= 100000){
disc = pre * 5.0 / 100.0;
comm = sum * 2.0 / 100.0;
}
else if(sum <= 200000){
disc = pre * 8.0 / 100.0;
comm = sum * 3.0 / 100.0;
}
else if(sum <= 500000){
disc = pre * 10.0 / 100.0;
comm = sum * 5.0 / 100.0;
}
else{
disc = pre * 15.0 / 100.0;
comm = sum * 7.5 / 100.0;
}
System.out.println("Name of the policy holder: " + name);
System.out.println("Sum assured: " + sum);
System.out.println("Premium: " + pre);
System.out.println("Discount on the first premium: " + disc);
System.out.println("Commission of the agent: " + comm);
}
}
Variable Description Table
Program Explanation
Output
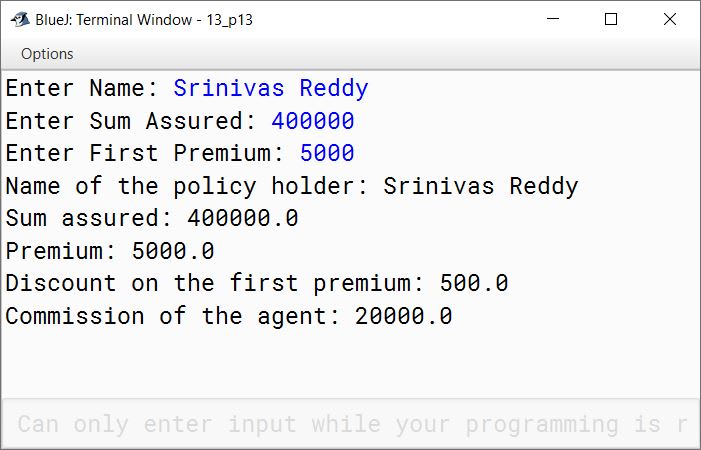
Answered By
70 Likes
Related Questions
An employee wants to deposit certain sum of money under 'Term Deposit' scheme in Syndicate Bank. The bank has provided the tariff of the scheme, which is given below:
No. of Days Rate of Interest Up to 180 days 5.5% 181 to 364 days 7.5% Exact 365 days 9.0% More than 365 days 8.5% Write a program to calculate the maturity amount taking the sum and number of days as inputs.
Using a switch case statement, write a menu driven program to convert a given temperature from Fahrenheit to Celsius and vice-versa. For an incorrect choice, an appropriate message should be displayed.
Hint: c = 5/9(f-32) and f=1.8c+32
A company announces revised Dearness Allowance (DA) and Special Allowances (SA) for their employees as per the tariff given below:
Basic Dearness Allowance (DA) Special Allowance (SA) Up to ₹ 10,000 10% 5% ₹ 10,001 - ₹ 20,000 12% 8% ₹ 20,001 - ₹ 30,000 15% 10% ₹ 30,001 and above 20% 12% Write a program to accept name and Basic Salary (BS) of an employee. Calculate and display gross salary.
Gross Salary = Basic + Dearness Allowance + Special Allowance
Print the information in the given format:
Name Basic DA Spl. Allowance Gross Salary
xxx xxx xxx xxx xxxGiven below is a hypothetical table showing rate of income tax for an India citizen, who is below or up to 60 years.
Taxable income (TI) in ₹ Income Tax in ₹ Up to ₹ 2,50,000 Nil More than ₹ 2,50,000 and less than or equal to ₹ 5,00,000 (TI - 1,60,000) * 10% More than ₹ 5,00,000 and less than or equal to ₹ 10,00,000 (TI - 5,00,000) * 20% + 34,000 More than ₹ 10,00,000 (TI - 10,00,000) * 30% + 94,000 Write a program to input the name, age and taxable income of a person. If the age is more than 60 years then display the message "Wrong Category". If the age is less than or equal to 60 years then compute and display the income tax payable along with the name of tax payer, as per the table given above.