Computer Applications
Given below is a hypothetical table showing rate of income tax for an India citizen, who is below or up to 60 years.
Taxable income (TI) in ₹ | Income Tax in ₹ |
---|---|
Up to ₹ 2,50,000 | Nil |
More than ₹ 2,50,000 and less than or equal to ₹ 5,00,000 | (TI - 1,60,000) * 10% |
More than ₹ 5,00,000 and less than or equal to ₹ 10,00,000 | (TI - 5,00,000) * 20% + 34,000 |
More than ₹ 10,00,000 | (TI - 10,00,000) * 30% + 94,000 |
Write a program to input the name, age and taxable income of a person. If the age is more than 60 years then display the message "Wrong Category". If the age is less than or equal to 60 years then compute and display the income tax payable along with the name of tax payer, as per the table given above.
Java
Java Conditional Stmts
115 Likes
Answer
import java.util.Scanner;
public class KboatIncomeTax
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Name: ");
String name = in.nextLine();
System.out.print("Enter age: ");
int age = in.nextInt();
System.out.print("Enter taxable income: ");
double ti = in.nextDouble();
double tax = 0.0;
if (age > 60) {
System.out.print("Wrong Category");
}
else {
if (ti <= 250000)
tax = 0;
else if (ti <= 500000)
tax = (ti - 160000) * 10 / 100;
else if (ti <= 1000000)
tax = 34000 + ((ti - 500000) * 20 / 100);
else
tax = 94000 + ((ti - 1000000) * 30 / 100);
}
System.out.println("Name: " + name);
System.out.println("Tax Payable: " + tax);
}
}
Variable Description Table
Program Explanation
Output
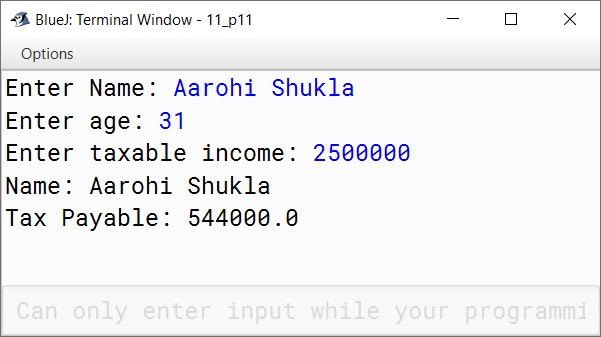
Answered By
51 Likes
Related Questions
Mr. Kumar is an LIC agent. He offers discount to his policy holders on the annual premium. However, he also gets commission on the sum assured as per the given tariff.
Sum Assured Discount Commission Up to ₹ 1,00,000 5% 2% ₹ 1,00,001 and up to ₹ 2,00,000 8% 3% ₹ 2,00,001 and up to ₹ 5,00,000 10% 5% More than ₹ 5,00,000 15% 7.5% Write a program to input name of the policy holder, the sum assured and first annual premium. Calculate the discount of the policy holder and the commission of the agent. The program displays all the details as:
Name of the policy holder :
Sum assured :
Premium :
Discount on the first premium :
Commission of the agent :An employee wants to deposit certain sum of money under 'Term Deposit' scheme in Syndicate Bank. The bank has provided the tariff of the scheme, which is given below:
No. of Days Rate of Interest Up to 180 days 5.5% 181 to 364 days 7.5% Exact 365 days 9.0% More than 365 days 8.5% Write a program to calculate the maturity amount taking the sum and number of days as inputs.
A cloth showroom has announced festival discounts and the gifts on the purchase of items, based on the total cost as given below:
Total Cost Discount Gift Up to ₹ 2,000 5% Calculator ₹ 2,001 to ₹ 5,000 10% School Bag ₹ 5,001 to ₹ 10,000 15% Wall Clock Above ₹ 10,000 20% Wrist Watch Write a program to input the total cost. Compute and display the amount to be paid by the customer along with the gift.
A Pre-Paid taxi charges from the passenger as per the tariff given below:
Distance Rate Up to 5 km ₹ 100 For the next 10 km ₹ 10/km For the next 10 km ₹ 8/km More than 25 km ₹ 5/km Write a program to input the distance covered and calculate the amount paid by the passenger. The program displays the printed bill with the details given below:
Taxi No. :
Distance covered :
Amount :