Computer Applications
In an election, there are two candidates X and Y. On the election day, 80% of the voters go for polling, out of which 60% vote for X. Write a program to take the number of voters as input and calculate:
- number of votes received by X
- number of votes received by Y
Java
Input in Java
100 Likes
Answer
import java.util.Scanner;
public class KboatPolling
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the number of voters: ");
long totalVoters = in.nextLong();
long votedVoters = Math.round(0.8 * totalVoters);
long xVotes = Math.round(0.6 * votedVoters);
long yVotes = votedVoters - xVotes;
System.out.println("Total Votes: " + votedVoters);
System.out.println("Votes of X: " + xVotes);
System.out.println("Votes of Y: " + yVotes);
}
}
Variable Description Table
Program Explanation
Output
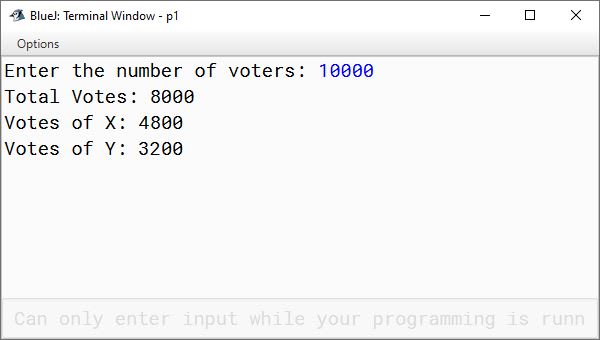
Answered By
40 Likes
Related Questions
What are the different types of errors that take place during the execution of a program?
Give two differences between Syntax error and Logical error.
A shopkeeper offers 10% discount on the printed price of a mobile phone. However, a customer has to pay 9% GST on the remaining amount. Write a program in Java to calculate the amount to be paid by the customer taking printed price as an input.
A man spends (1/2) of his salary on food, (1/15) on rent, (1/10) on miscellaneous activities. Rest of the salary is his saving. Write a program to calculate and display the following:
- money spent on food
- money spent on rent
- money spent on miscellaneous activities
- money saved
Take the salary as an input.