Computer Applications
A man spends (1/2) of his salary on food, (1/15) on rent, (1/10) on miscellaneous activities. Rest of the salary is his saving. Write a program to calculate and display the following:
- money spent on food
- money spent on rent
- money spent on miscellaneous activities
- money saved
Take the salary as an input.
Java
Input in Java
64 Likes
Answer
import java.util.Scanner;
public class KboatSalary
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the Salary: ");
float salary = in.nextFloat();
float foodSpend = salary / 2;
float rentSpend = salary / 15;
float miscSpend = salary / 10;
float savings = salary - (foodSpend + rentSpend + miscSpend);
System.out.println("Money spent on food: " + foodSpend);
System.out.println("Money spent on rent: " + rentSpend);
System.out.println("Money spent on miscellaneous: " + miscSpend);
System.out.println("Money saved: " + savings);
}
}
Variable Description Table
Program Explanation
Output
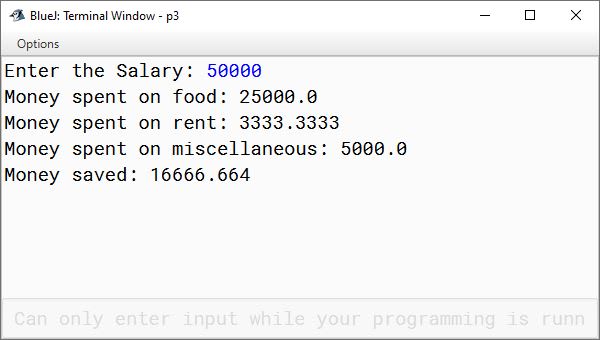
Answered By
32 Likes
Related Questions
A shopkeeper offers 10% discount on the printed price of a mobile phone. However, a customer has to pay 9% GST on the remaining amount. Write a program in Java to calculate the amount to be paid by the customer taking printed price as an input.
Write a program to input time in seconds. Display the time after converting them into hours, minutes and seconds.
Sample Input: Time in seconds: 5420
Sample Output: 1 Hour 30 Minutes 20 Seconds
In an election, there are two candidates X and Y. On the election day, 80% of the voters go for polling, out of which 60% vote for X. Write a program to take the number of voters as input and calculate:
- number of votes received by X
- number of votes received by Y
The driver took a drive to a town 240 km at a speed of 60 km/h. Later in the evening, he drove back at 20 km/h less than the usual speed. Write a program to calculate:
- the total time taken by the driver
- the average speed during the whole journey
[Hint: average speed = total distance / total time]