Computer Applications
Design a class to overload the method display(…..) as follows:
- void display(int num) — checks and prints whether the number is a perfect square or not.
- void display(String str, char ch) — checks and prints if the word str contains the letter ch or not.
- void display(String str) — checks and prints the number of special characters present in the word str.
Write a suitable main( ) function.
Java
User Defined Methods
31 Likes
Answer
public class KboatDisplay
{
public void display(int num) {
double sroot = Math.sqrt(num);
double diff = sroot - Math.floor(sroot);
if (diff == 0)
System.out.println(num + " is a perfect square");
else
System.out.println(num + " is not a perfect square");
}
public void display(String str, char ch) {
int idx = str.indexOf(ch);
if (idx == -1)
System.out.println(ch + " not found");
else
System.out.println(ch + " found");
}
public void display(String str) {
int count = 0;
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (!Character.isLetterOrDigit(ch) &&
!Character.isWhitespace(ch)) {
count++;
}
}
System.out.println("Number of special characters = " + count);
}
public static void main(String args[]) {
KboatDisplay obj = new KboatDisplay();
obj.display(18);
obj.display("ICSE Computer Applications", 't');
obj.display("https://www.knowledgeboat.com/");
}
}
Variable Description Table
Program Explanation
Output
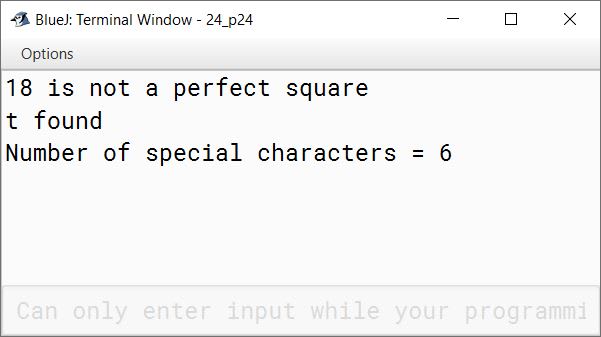
Answered By
13 Likes
Related Questions
Design a class to overload a method series( ) as follows:
- double series(double n) with one double argument and returns the sum of the series.
sum = (1/1) + (1/2) + (1/3) + ………. + (1/n) - double series(double a, double n) with two double arguments and returns the sum of the series.
sum = (1/a2) + (4/a5) + (7/a8) + (10/a11) + ………. to n terms
- double series(double n) with one double argument and returns the sum of the series.
Design a class to overload the method display(…..) as follows:
- void display(String str, char ch) — checks whether the word str contains the letter ch at the beginning as well as at the end or not. If present, print 'Special Word' otherwise print 'No special word'.
- void display(String str1, String str2) — checks and prints whether both the words are equal or not.
- void display(String str, int n) — prints the character present at nth position in the word str.
Write a suitable main() method.
Design a class to overload a method volume( ) as follows:
- double volume(double r) — with radius (r) as an argument, returns the volume of sphere using the formula:
V = (4/3) * (22/7) * r * r * r - double volume(double h, double r) — with height(h) and radius(r) as the arguments, returns the volume of a cylinder using the formula:
V = (22/7) * r * r * h - double volume(double 1, double b, double h) — with length(l), breadth(b) and height(h) as the arguments, returns the volume of a cuboid using the formula:
V = l*b*h ⇒ (length * breadth * height)
- double volume(double r) — with radius (r) as an argument, returns the volume of sphere using the formula:
Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.