Computer Applications
Design a class to overload a method series( ) as follows:
- double series(double n) with one double argument and returns the sum of the series.
sum = (1/1) + (1/2) + (1/3) + ………. + (1/n) - double series(double a, double n) with two double arguments and returns the sum of the series.
sum = (1/a2) + (4/a5) + (7/a8) + (10/a11) + ………. to n terms
Java
User Defined Methods
ICSE 2013
214 Likes
Answer
public class KboatSeries
{
double series(double n) {
double sum = 0;
for (int i = 1; i <= n; i++) {
double term = 1.0 / i;
sum += term;
}
return sum;
}
double series(double a, double n) {
double sum = 0;
int x = 1;
for (int i = 1; i <= n; i++) {
int e = x + 1;
double term = x / Math.pow(a, e);
sum += term;
x += 3;
}
return sum;
}
public static void main(String args[]) {
KboatSeries obj = new KboatSeries();
System.out.println("First series sum = " + obj.series(5));
System.out.println("Second series sum = " + obj.series(3, 8));
}
}
Variable Description Table
Program Explanation
Output
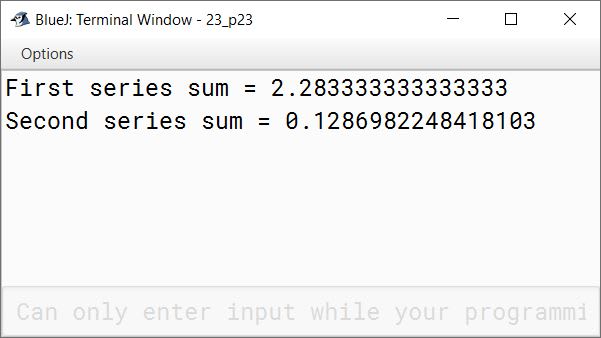
Answered By
58 Likes
Related Questions
Design a class to overload the method display(…..) as follows:
- void display(int num) — checks and prints whether the number is a perfect square or not.
- void display(String str, char ch) — checks and prints if the word str contains the letter ch or not.
- void display(String str) — checks and prints the number of special characters present in the word str.
Write a suitable main( ) function.
Design a class to overload the method display(…..) as follows:
- void display(String str, char ch) — checks whether the word str contains the letter ch at the beginning as well as at the end or not. If present, print 'Special Word' otherwise print 'No special word'.
- void display(String str1, String str2) — checks and prints whether both the words are equal or not.
- void display(String str, int n) — prints the character present at nth position in the word str.
Write a suitable main() method.
Design a class overloading a method calculate() as follows:
- void calculate(int m, char ch) with one integer argument and one character argument. It checks whether the integer argument is divisible by 7 or not, if ch is 's', otherwise, it checks whether the last digit of the integer argument is 7 or not.
- void calculate(int a, int b, char ch) with two integer arguments and one character argument. It displays the greater of integer arguments if ch is 'g' otherwise, it displays the smaller of integer arguments.
Design a class to overload a method compare( ) as follows:
- void compare(int, int) — to compare two integers values and print the greater of the two integers.
- void compare(char, char) — to compare the numeric value of two characters and print with the higher numeric value.
- void compare(String, String) — to compare the length of the two strings and print the longer of the two.