Computer Applications
Design a class to overload a function num_calc() as follows:
- void num_calc(int mini, char ch) with one integer argument and one character argument, computes the square of integer argument if choice ch is 's' otherwise finds its cube.
- void num_calc (int a, int b, char ch) with two integer arguments and one character argument. It computes the product of integer arguments if ch is 'p' else adds the integers.
- void num_calc (String s1, String s2) with two string arguments, which prints whether the strings are equal or not.
Java
Java String Handling
16 Likes
Answer
import java.util.Scanner;
public class KboatChoiceOverload
{
void num_calc(int mini, char ch) {
if (ch == 's') {
long sq = mini * mini;
System.out.println("Square = " + sq );
}
else {
long cube = mini * mini * mini;
System.out.println("Cube = " + cube);
}
}
void num_calc(int a, int b, char ch) {
if (ch == 'p') {
long prod = a * b;
System.out.println("Product = " + prod );
}
else {
long sum = a + b;
System.out.println("Sum = " + sum);
}
}
void num_calc(String s1, String s2) {
if(s1.equals(s2))
System.out.println("Strings are equal");
else
System.out.println("Strings are not equal");
}
}
Output
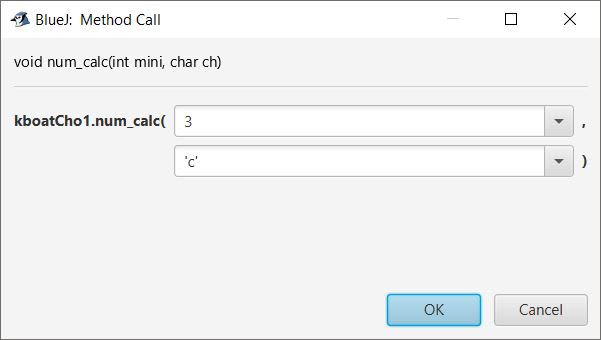
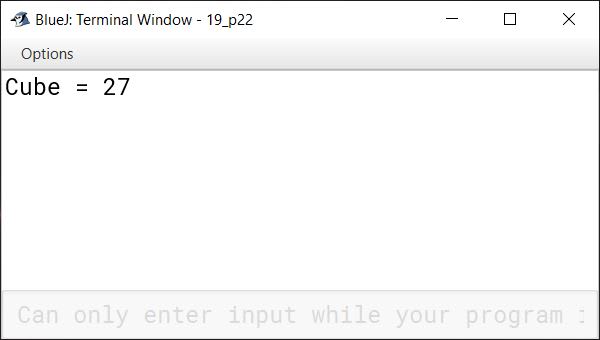
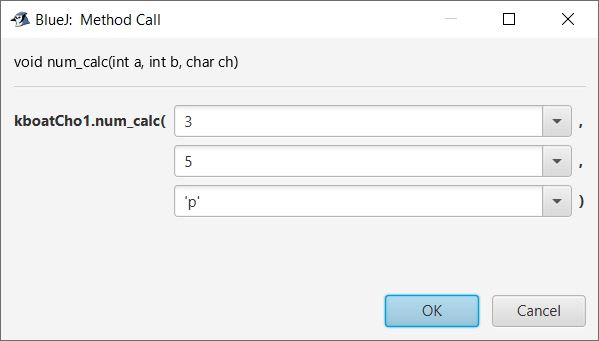
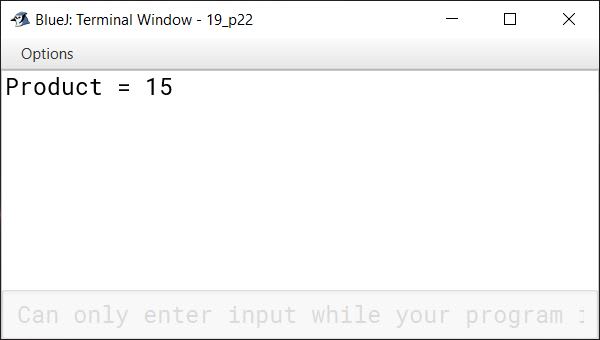
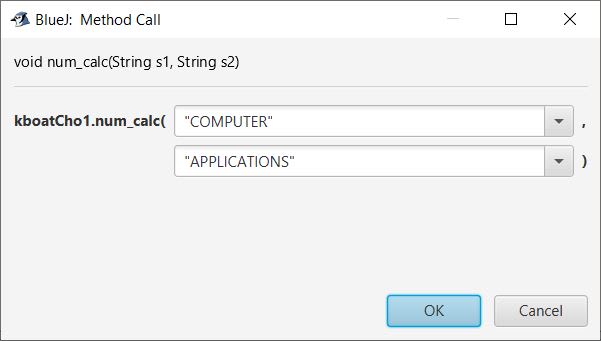
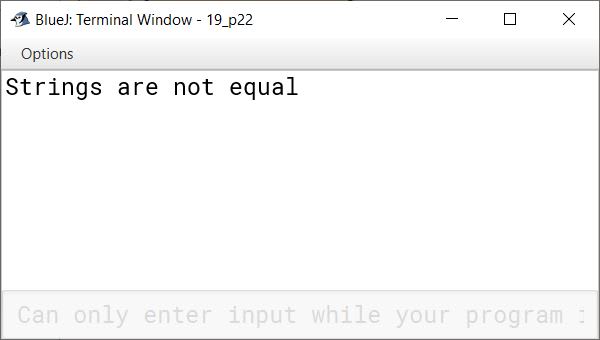
Answered By
3 Likes
Related Questions
Design a class RailwayTicket with following description:
Class name : RailwayTicket
Data Members Purpose String name To store the name of the customer String coach To store the type of coach customer wants to travel long mob no To store customer's mobile number int amt To store basic amount of ticket int totalamt To store the amount to be paid after updating the original amount Member Methods Purpose void accept() To take input for name, coach, mobile number and amount void update() To update the amount as per the coach selected (extra amount to be added in the amount as per the table below) void display() To display all details of a customer such as name, coach, total amount and mobile number Type of Coaches Amount First_AC ₹700 Second_AC ₹500 Third_AC ₹250 Sleeper None Write a main method to create an object of the class and call the above member methods.
Design a class to overload a function Joystring( ) as follows:
- void Joystring(String s, char ch1, char ch2) with one string argument and two character arguments that replaces the character argument ch1 with the character argument ch2 in the given String s and prints the new string.
Example:
Input value of s = "TECHNALAGY"
ch1 = 'A'
ch2 = 'O'
Output: "TECHNOLOGY" - void Joystring(String s) with one string argument that prints the position of the first space and the last space of the given String s.
Example:
Input value of s = "Cloud computing means Internet based computing"
Output:
First index: 5
Last Index: 36 - void Joystring(String s1, String s2) with two string arguments that combines the two strings with a space between them and prints the resultant string.
Example:
Input value of s1 = "COMMON WEALTH"
Input value of s2 = "GAMES"
Output: COMMON WEALTH GAMES
(Use library functions)
- void Joystring(String s, char ch1, char ch2) with one string argument and two character arguments that replaces the character argument ch1 with the character argument ch2 in the given String s and prints the new string.
Design a class to overload a function compare( ) as follows:
- void compare(int, int) - to compare two integer values and print the greater of the two integers.
- void compare(char, char) - to compare the numeric value of two characters with higher numeric value.
- void compare(String, String) - to compare the length of the two strings and print the longer of the two.
Design a class to overload a function check( ) as follows:
void check (String str , char ch ) — to find and print the frequency of a character in a string.
Example:
Input:
str = "success"
ch = 's'
Output:
number of s present is = 3void check(String s1) — to display only vowels from string s1, after converting it to lower case.
Example:
Input:
s1 ="computer"
Output : o u e