Computer Applications
Define a class Arrange described as below:
Data members/instance variables:
- String str (a word)
- String i
- int p (to store the length of the word)
- char ch;
Member Methods:
- A parameterised constructor to initialize the data member
- To accept the word
- To arrange all the alphabets of word in ascending order of their ASCII values without using the sorting technique
- To display the arranged alphabets.
Write a main method to create an object of the class and call the above member methods.
Java
Java Constructors
52 Likes
Answer
import java.util.Scanner;
public class Arrange
{
private String str;
private String i;
private int p;
private char ch;
public Arrange(String s) {
str = s;
i = "";
p = s.length();
ch = 0;
}
public void rearrange() {
for (int a = 65; a <= 90; a++) {
for (int j = 0; j < p; j++) {
ch = str.charAt(j);
if (a == Character.toUpperCase(ch))
i += ch;
}
}
}
public void display() {
System.out.println("Alphabets in ascending order:");
System.out.println(i);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a word: ");
String word = in.nextLine();
Arrange obj = new Arrange(word);
obj.rearrange();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
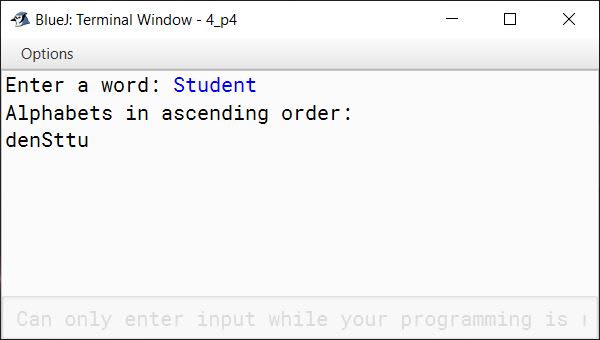
Answered By
19 Likes
Related Questions
Write a program by using a class with the following specifications:
Class name — Calculate
Instance variables:
- int num
- int f
- int rev
Member Methods:
- Calculate(int n) — to initialize num with n, f and rev with 0 (zero)
- int prime() — to return 1, if number is prime
- int reverse() — to return reverse of the number
- void display() — to check and print whether the number is a prime palindrome or not
Write a program by using a class in Java with the following specifications:
Class name — Stringop
Data members:
- String str
Member functions:
- Stringop() — to initialize str with NULL
- void accept() — to input a sentence
- void encode() — to replace and print each character of the string with the second next character in the ASCII table. For example, A with C, B with D and so on
- void print() — to print each word of the String in a separate line
An electronics shop has announced a special discount on the purchase of Laptops as given below:
Category Discount on Laptop Up to ₹25,000 5.0% ₹25,001 - ₹50,000 7.5% ₹50,001 - ₹1,00,000 10.0% More than ₹1,00,000 15.0% Define a class Laptop described as follows:
Data members/instance variables:
- name
- price
- dis
- amt
Member Methods:
- A parameterised constructor to initialize the data members
- To accept the details (name of the customer and the price)
- To compute the discount
- To display the name, discount and amount to be paid after discount.
Write a main method to create an object of the class and call the member methods.
The population of a country in a particular year can be calculated by:
p*(1+r/100) at the end of year 2000, where p is the initial population and r is the
growth rate.Write a program by using a class to find the population of the country at the end of each year from 2001 to 2007. The Class has the following specifications:
Class name — Population
Data Members — float p,r
Member Methods:
- Population(int a,int b) — Constructor to initialize p and r with a and b respectively.
- void print() — to calculate and print the population of each year from 2001 to 2007.