Computer Applications
The population of a country in a particular year can be calculated by:
p*(1+r/100) at the end of year 2000, where p is the initial population and r is the
growth rate.
Write a program by using a class to find the population of the country at the end of each year from 2001 to 2007. The Class has the following specifications:
Class name — Population
Data Members — float p,r
Member Methods:
- Population(int a,int b) — Constructor to initialize p and r with a and b respectively.
- void print() — to calculate and print the population of each year from 2001 to 2007.
Java
Java Constructors
50 Likes
Answer
import java.util.Scanner;
public class Population
{
private float p;
private float r;
public Population(float a, float b)
{
p = a;
r = b;
}
public void print() {
float q = p;
for (int y = 2001; y <= 2007; y++) {
q = q * (1 + r / 100);
System.out.println("Population in " + y + ": " + q);
}
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter population in the year 2000: ");
float x = in.nextFloat();
System.out.print("Enter growth rate: ");
float y = in.nextFloat();
Population obj = new Population(x,y);
obj.print();
}
}
Variable Description Table
Program Explanation
Output
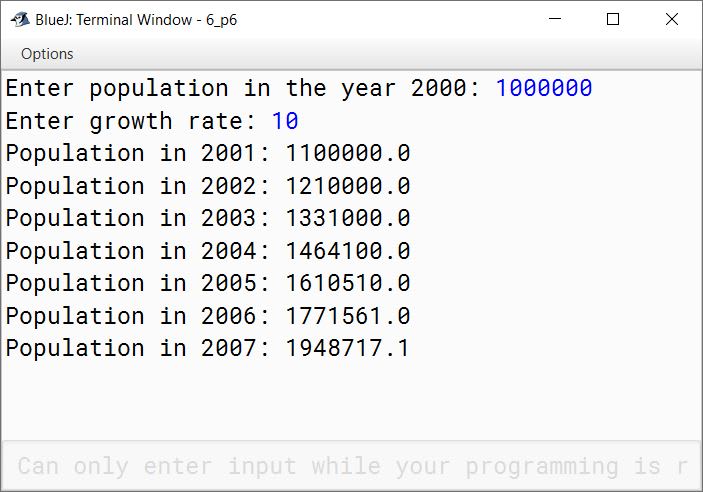
Answered By
22 Likes
Related Questions
Define a class Arrange described as below:
Data members/instance variables:
- String str (a word)
- String i
- int p (to store the length of the word)
- char ch;
Member Methods:
- A parameterised constructor to initialize the data member
- To accept the word
- To arrange all the alphabets of word in ascending order of their ASCII values without using the sorting technique
- To display the arranged alphabets.
Write a main method to create an object of the class and call the above member methods.
Write a program in Java to find the roots of a quadratic equation ax2+bx+c=0 with the following specifications:
Class name — Quad
Data Members — float a,b,c,d (a,b,c are the co-efficients & d is the discriminant), r1 and r2 are the roots of the equation.
Member Methods:
- quad(int x,int y,int z) — to initialize a=x, b=y, c=z, d=0
- void calculate() — Find d=b2-4ac
If d < 0 then print "Roots not possible" otherwise find and print:
r1 = (-b + √d) / 2a
r2 = (-b - √d) / 2aWrite a program by using a class in Java with the following specifications:
Class name — Stringop
Data members:
- String str
Member functions:
- Stringop() — to initialize str with NULL
- void accept() — to input a sentence
- void encode() — to replace and print each character of the string with the second next character in the ASCII table. For example, A with C, B with D and so on
- void print() — to print each word of the String in a separate line
Define a class named FruitJuice with the following description:
Data Members Purpose int product_code stores the product code number String flavour stores the flavour of the juice (e.g., orange, apple, etc.) String pack_type stores the type of packaging (e.g., tera-pack, PET bottle, etc.) int pack_size stores package size (e.g., 200 mL, 400 mL, etc.) int product_price stores the price of the product Member Methods Purpose FruitJuice() constructor to initialize integer data members to 0 and string data members to "" void input() to input and store the product code, flavour, pack type, pack size and product price void discount() to reduce the product price by 10 void display() to display the product code, flavour, pack type, pack size and product price