Computer Applications
Declare a single dimensional array of size 28 to store daily temperatures for the month of February. Using this structure, write a program to find:
- The hottest day of the month
- The coldest day of the month
- The average temperature of the month
Answer
import java.util.Scanner;
public class KboatFebTemp
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
double febTemp[] = new double[28];
int n = febTemp.length;
System.out.println("Enter Feb daily temperatures:");
for (int i = 0; i < n; i++) {
febTemp[i] = in.nextDouble();
}
double sum = 0.0;
int low = 0, high = 0;
for (int i = 0; i < n; i++) {
if (febTemp[i] < febTemp[low])
low = i;
if (febTemp[i] > febTemp[high])
high = i;
sum += febTemp[i];
}
double avg = sum / n;
System.out.println("Hottest day = " + (high + 1));
System.out.println("Coldest day = " + (low + 1));
System.out.println("Average Temperature = " + avg);
}
}
Output
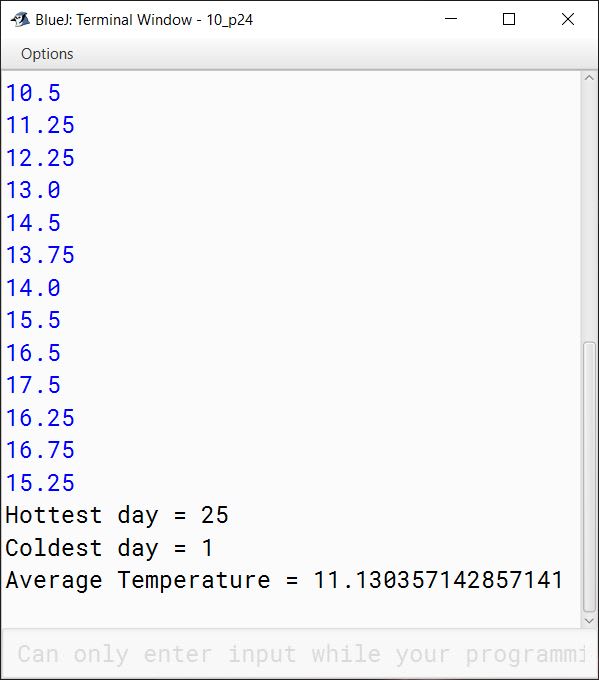
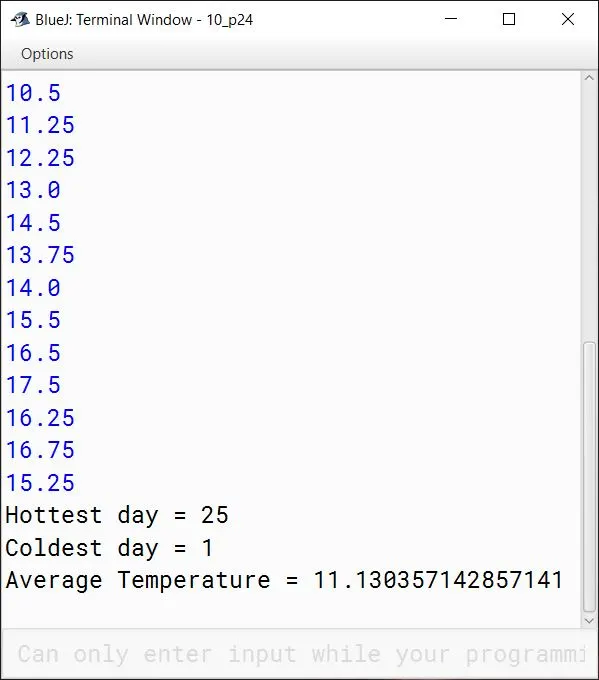
Related Questions
Write a program to store 6 elements in an array P and 4 elements in an array Q. Now, produce a third array R, containing all the elements of array P and Q. Display the resultant array.
Input Input Output P[ ] Q[ ] R[ ] 4 19 4 6 23 6 1 7 1 2 8 2 3 3 10 10 19 23 7 8 The annual examination result of 50 students in a class is tabulated in a Single Dimensional Array (SDA) is as follows:
Roll No. Subject A Subject B Subject C ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. Write a program to read the data, calculate and display the following:
(a) Average marks obtained by each student.
(b) Print the roll number and the average marks of the students whose average is above. 80.
(c) Print the roll number and the average marks of the students whose average is below 40.Declare a two-dimensional array with 4 rows and 7 columns to store daily temperatures for the month of February. Using this structure, write a program to find:
- The hottest day of the month
- The coldest day of the month
- The average temperature of the month
The weekly hours of all employees of ABC Consulting Ltd. are stored in a two-dimensional array. Each row records an employee's 7-day work hours with seven columns. For example, the following array stores the work hours of five employees. Write a program that displays employees and their total hours in decreasing order of the total hours.
S. No. Mon Tue Wed Thu Fri Sat Sun Employee 0 8 5 1 11 1 0 0 Employee 1 11 6 0 2 2 2 4 Employee 2 4 5 4 10 4 3 1 Employee 3 4 7 3 12 6 2 0 Employee 4 3 8 3 2 4 4 0