Computer Applications
The weekly hours of all employees of ABC Consulting Ltd. are stored in a two-dimensional array. Each row records an employee's 7-day work hours with seven columns. For example, the following array stores the work hours of five employees. Write a program that displays employees and their total hours in decreasing order of the total hours.
S. No. | Mon | Tue | Wed | Thu | Fri | Sat | Sun |
---|---|---|---|---|---|---|---|
Employee 0 | 8 | 5 | 1 | 11 | 1 | 0 | 0 |
Employee 1 | 11 | 6 | 0 | 2 | 2 | 2 | 4 |
Employee 2 | 4 | 5 | 4 | 10 | 4 | 3 | 1 |
Employee 3 | 4 | 7 | 3 | 12 | 6 | 2 | 0 |
Employee 4 | 3 | 8 | 3 | 2 | 4 | 4 | 0 |
Java
Java Arrays
9 Likes
Answer
import java.util.Scanner;
public class KboatABCConsulting
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter total number of employees: ");
int numEmps = in.nextInt();
int workHours[][] = new int[numEmps][7];
int empArr[] = new int[numEmps];
int hours[] = new int[numEmps];
//Enter employee hours in 2D array
for (int i = 0; i < numEmps; i++) {
System.out.println("Enter hours for Employee " + i);
for (int j = 0; j < 7; j++) {
workHours[i][j] = in.nextInt();
}
}
//Calculate total hours for each employee
for (int i = 0; i < numEmps; i++) {
int totalHours = 0;
for (int j = 0; j < 7; j++) {
totalHours += workHours[i][j];
}
empArr[i] = i;
hours[i] = totalHours;
}
//Sort total hours hours in decreasing order
for (int i = 0; i < numEmps - 1; i++) {
int index = i;
for (int j = i + 1; j < numEmps; j++) {
if (hours[j] > hours[index])
index = j;
}
int t = hours[i];
hours[i] = hours[index];
hours[index] = t;
t = empArr[i];
empArr[i] = empArr[index];
empArr[index] = t;
}
//Display sorted total hours
for (int i = 0; i < numEmps; i++)
System.out.println("Employee " + empArr[i]
+ "\t" + hours[i]);
}
}
Output
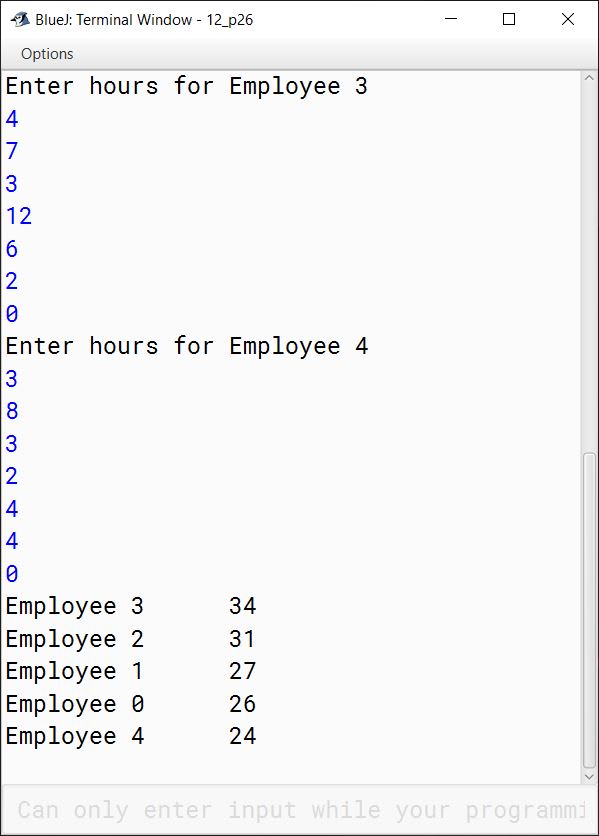
Answered By
2 Likes
Related Questions
The annual examination result of 50 students in a class is tabulated in a Single Dimensional Array (SDA) is as follows:
Roll No. Subject A Subject B Subject C ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. Write a program to read the data, calculate and display the following:
(a) Average marks obtained by each student.
(b) Print the roll number and the average marks of the students whose average is above. 80.
(c) Print the roll number and the average marks of the students whose average is below 40.Declare a single dimensional array of size 28 to store daily temperatures for the month of February. Using this structure, write a program to find:
- The hottest day of the month
- The coldest day of the month
- The average temperature of the month
Declare a two-dimensional array with 4 rows and 7 columns to store daily temperatures for the month of February. Using this structure, write a program to find:
- The hottest day of the month
- The coldest day of the month
- The average temperature of the month
Write a program that computes the standard deviation of N real numbers. The standard deviation s is computed according to:
1 - \overset{-}{x})^2 + (x2 - \overset{-}{x})^2 + … + (x_N - \overset{-}{x})^2}{N}}
The variable is the average of N input values 1 through . The program first prompts the user for N and then declares an array of size N.