Computer Applications
An Electricity Board charges for electricity per month from their consumers according to the units consumed. The tariff is given below:
Units Consumed | Charges |
---|---|
Up to 200 units | ₹3.80/unit |
More than 200 units and up to 300 units | ₹4.40/unit |
More than 300 units and up to 400 units | ₹5.10/unit |
More than 400 units | ₹5.80/unit |
Write a program to calculate the electricity bill taking consumer's name and units consumed as inputs. Display the output.
Java
Java Conditional Stmts
115 Likes
Answer
import java.util.Scanner;
public class KboatElectricBill
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Consumer Name: ");
String name = in.nextLine();
System.out.print("Enter Unit's Consumed: ");
int units = in.nextInt();
double amt = 0.0;
if (units <= 200)
amt = units * 3.8;
else if (units <= 300)
amt = (200 * 3.8) + ((units - 200) * 4.4);
else if (units <= 400)
amt = (200 * 3.8) + (100 * 4.4) + ((units - 300) * 5.1);
else
amt = (200 * 3.8) + (100 * 4.4) + (100 * 5.1) + ((units - 400) * 5.8);
System.out.println("Consumer Name: " + name);
System.out.println("Units Consumed: " + units);
System.out.println("Bill Amount: " + amt);
}
}
Variable Description Table
Program Explanation
Output
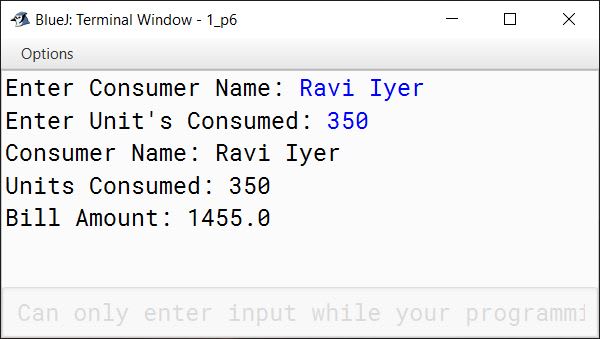
Answered By
48 Likes
Related Questions
Give the output of the snippet:
int a=10,b=12; if(a==10&&b<=12) a--; else ++b; System.out.println(a + "and" +b);
Give the output of the snippet:
int p = 9; while (p<=15) { p++; if(p== 10) continue; System.out.println(p); }
The equivalent resistance of series and parallel connections of two resistances are given by the formula:
(a) R1 = r1 + r2 (Series)
(b) R2 = (r1 * r2) / (r1 + r2) (Parallel)
Using a switch case statement, write a program to enter the value of r1 and r2. Calculate and display the equivalent resistances accordingly.
Write a program to display the first ten terms of the series:
5, 10, 17, --------------