Computer Applications
A courier company charges differently for 'Ordinary' booking and 'Express' booking based on the weight of the parcel as per the tariff given below:
Weight of parcel | Ordinary booking | Express booking |
---|---|---|
Up to 100 gm | ₹80 | ₹100 |
101 to 500 gm | ₹150 | ₹200 |
501 gm to 1000 gm | ₹210 | ₹250 |
More than 1000 gm | ₹250 | ₹300 |
Write a program to input weight of a parcel and type of booking (`O' for ordinary and 'E' for express). Calculate and print the charges accordingly.
Java
Java Conditional Stmts
82 Likes
Answer
import java.util.Scanner;
public class KboatCourierCompany
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter weight of parcel: ");
double wt = in.nextDouble();
System.out.print("Enter type of booking: ");
char type = in.next().charAt(0);
double charge = 0;
if (wt <= 0)
charge = 0;
else if (wt <= 100 && type == 'O')
charge = 80;
else if (wt <= 100 && type == 'E')
charge = 100;
else if (wt <= 500 && type == 'O')
charge = 150;
else if (wt <= 500 && type == 'E')
charge = 200;
else if (wt <= 1000 && type == 'O')
charge = 210;
else if (wt <= 1000 && type == 'E')
charge = 250;
else if (wt > 1000 && type == 'O')
charge = 250;
else if (wt > 1000 && type == 'E')
charge = 300;
System.out.println("Parcel charges = " + charge);
}
}
Variable Description Table
Program Explanation
Output
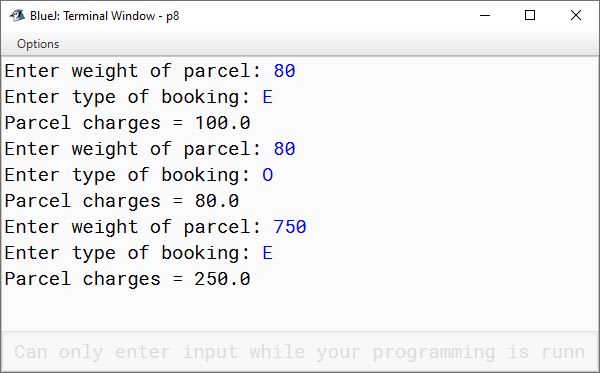
Answered By
34 Likes
Related Questions
A bank announces new rates for Term Deposit Schemes for their customers and Senior Citizens as given below:
Term Rate of Interest (General) Rate of Interest (Senior Citizen) Up to 1 year 7.5% 8.0% Up to 2 years 8.5% 9.0% Up to 3 years 9.5% 10.0% More than 3 years 10.0% 11.0% The 'senior citizen' rates are applicable to the customers whose age is 60 years or more. Write a program to accept the sum (p) in term deposit scheme, age of the customer and the term. The program displays the information in the following format:
Amount Deposited Term Age Interest earned Amount Paid xxx xxx xxx xxx xxx An ICSE school displays a notice on the school notice board regarding admission in Class XI for choosing stream according to marks obtained in English, Maths and Science in Class 10 Council Examination.
Marks obtained in different subjects Stream Eng, Maths and Science >= 80% Pure Science Eng and Science >= 80%, Maths >= 60% Bio. Science Eng, Maths and Science >= 60% Commerce Print the appropriate stream allotted to a candidate. Write a program to accept marks in English, Maths and Science from the console.
Write a program to input a number and check whether it is a perfect square or not. If the number is not a perfect square then find the least number to be added to input number, so that the resulting number is a perfect square.
Example 1:
Sample Input: 2025
√2025 = 45
Sample Output: It is a perfect square.
Example 2:
Sample Input: 1950
√1950 = 44.1588……..
Least number to be added = 452 - 1950 = 2025 - 1950 = 75Write a program that accepts three numbers from the user and displays them either in "Increasing Order" or in "Decreasing Order" as per the user's choice.
Choice 1: Ascending order
Choice 2: Descending orderSample Inputs: 394, 475, 296
Choice 2
Sample Output
First number : 475
Second number: 394
Third number : 296
The numbers are in decreasing order.