Computer Applications
A bank announces new rates for Term Deposit Schemes for their customers and Senior Citizens as given below:
Term | Rate of Interest (General) | Rate of Interest (Senior Citizen) |
---|---|---|
Up to 1 year | 7.5% | 8.0% |
Up to 2 years | 8.5% | 9.0% |
Up to 3 years | 9.5% | 10.0% |
More than 3 years | 10.0% | 11.0% |
The 'senior citizen' rates are applicable to the customers whose age is 60 years or more. Write a program to accept the sum (p) in term deposit scheme, age of the customer and the term. The program displays the information in the following format:
Amount Deposited | Term | Age | Interest earned | Amount Paid |
---|---|---|---|---|
xxx | xxx | xxx | xxx | xxx |
Java
Java Conditional Stmts
94 Likes
Answer
import java.util.Scanner;
public class KboatBankDeposit
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter sum: ");
double sum = in.nextDouble();
System.out.print("Enter age: ");
int age = in.nextInt();
System.out.print("Enter term: ");
double term = in.nextDouble();
double si = 0.0;
if (term <= 1 && age < 60)
si = (sum * 7.5 * term) / 100;
else if (term <= 1 && age >= 60)
si = (sum * 8.0 * term) / 100;
else if (term <= 2 && age < 60)
si = (sum * 8.5 * term) / 100;
else if (term <= 2 && age >= 60)
si = (sum * 9.0 * term) / 100;
else if (term <= 3 && age < 60)
si = (sum * 9.5 * term) / 100;
else if (term <= 3 && age >= 60)
si = (sum * 10.0 * term) / 100;
else if (term > 3 && age < 60)
si = (sum * 10.0 * term) / 100;
else if (term > 3 && age >= 60)
si = (sum * 11.0 * term) / 100;
double amt = sum + si;
System.out.println("Amount Deposited: " + sum);
System.out.println("Term: " + term);
System.out.println("Age: " + age);
System.out.println("Interest Earned: " + si);
System.out.println("Amount Paid: " + amt);
}
}
Variable Description Table
Program Explanation
Output
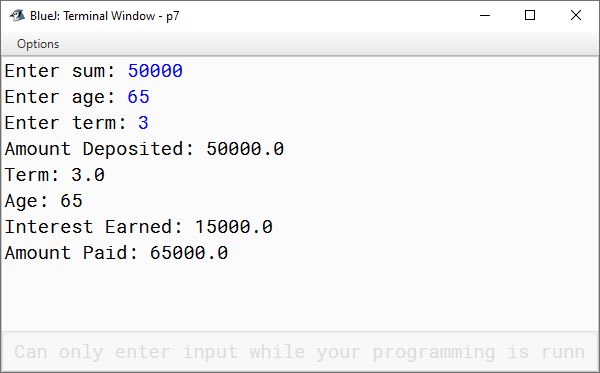
Answered By
46 Likes
Related Questions
An ICSE school displays a notice on the school notice board regarding admission in Class XI for choosing stream according to marks obtained in English, Maths and Science in Class 10 Council Examination.
Marks obtained in different subjects Stream Eng, Maths and Science >= 80% Pure Science Eng and Science >= 80%, Maths >= 60% Bio. Science Eng, Maths and Science >= 60% Commerce Print the appropriate stream allotted to a candidate. Write a program to accept marks in English, Maths and Science from the console.
A courier company charges differently for 'Ordinary' booking and 'Express' booking based on the weight of the parcel as per the tariff given below:
Weight of parcel Ordinary booking Express booking Up to 100 gm ₹80 ₹100 101 to 500 gm ₹150 ₹200 501 gm to 1000 gm ₹210 ₹250 More than 1000 gm ₹250 ₹300 Write a program to input weight of a parcel and type of booking (`O' for ordinary and 'E' for express). Calculate and print the charges accordingly.
An air-conditioned bus charges fare from the passengers based on the distance travelled as per the tariff given below:
Distance Travelled Fare Up to 10 km Fixed charge ₹80 11 km to 20 km ₹6/km 21 km to 30 km ₹5/km 31 km and above ₹4/km Design a program to input distance travelled by the passenger. Calculate and display the fare to be paid.
Write a program to input a number and check whether it is a perfect square or not. If the number is not a perfect square then find the least number to be added to input number, so that the resulting number is a perfect square.
Example 1:
Sample Input: 2025
√2025 = 45
Sample Output: It is a perfect square.
Example 2:
Sample Input: 1950
√1950 = 44.1588……..
Least number to be added = 452 - 1950 = 2025 - 1950 = 75