Computer Applications
You can multiply two numbers 'm' and 'n' by repeated addition method.
For example, 5 * 3 = 15 can be performed by adding 5 three times ⇒ 5 + 5 + 5 = 15
Similarly, successive subtraction of two numbers produces 'Quotient' and 'Remainder' when a number 'a' is divided by 'b' (a>b).
For example, 5/2 ⇒ Quotient = 2 and Remainder = 1
Follow steps shown below:
Process | Result | Counter |
---|---|---|
5 - 2 | 3 | 1 |
3 - 2 | 1 | 2 |
Sample Output: The last counter value represents 'Quotient' ⇒ 2
The last result value represents 'Remainder' ⇒ 1
Write a program to accept two numbers. Perform multiplication and division of the numbers as per the process shown above by using switch case statement.
Java
Java Iterative Stmts
19 Likes
Answer
import java.util.Scanner;
public class KboatArithmetic
{
public void computeResult() {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
System.out.println("1. Multiplication");
System.out.println("2. Division");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
int rMul = 0;
for (int i = 1; i <= b; i++) {
rMul += a;
}
System.out.println("Multiplication result = " + rMul);
break;
case 2:
int count = 0, t = a;
while (t > b) {
t -= b;
count++;
}
System.out.println("Divison Result");
System.out.println("Quotient = " + count);
System.out.println("Remainder = " + t);
break;
default:
System.out.println("Incorrect Choice");
break;
}
}
}
Variable Description Table
Program Explanation
Output
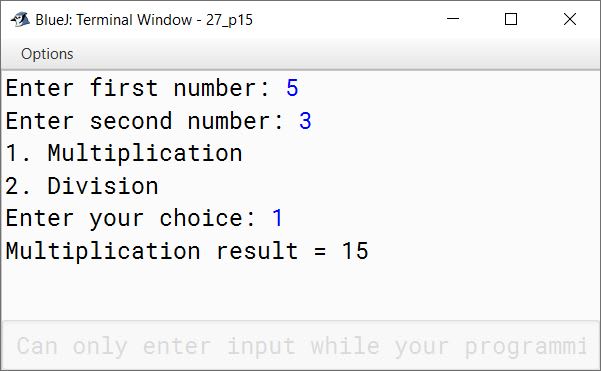
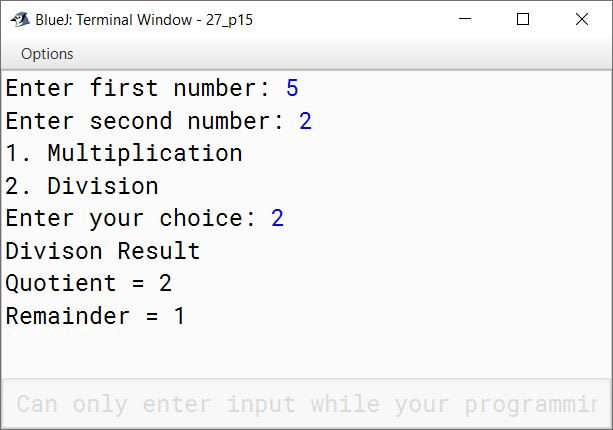
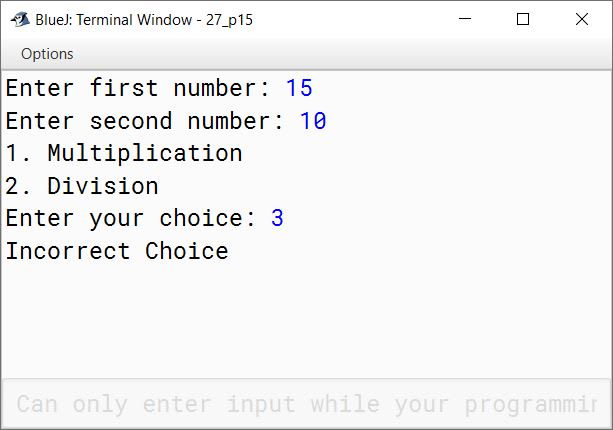
Answered By
6 Likes
Related Questions
Write a program to input a number and check whether it is a Harshad Number or not. [A number is said to be Harshad number, if it is divisible by the sum of its digits. The program displays the message accordingly.]
For example;
Sample Input: 132
Sum of digits = 6 and 132 is divisible by 6.
Output: It is a Harshad Number.
Sample Input: 353
Output: It is not a Harshad Number.Write a program to display all Pronic numbers in the range from 1 to n.
Hint: A Pronic number is a number which is the product of two consecutive numbers in the form of n * (n + 1).
For example;
2, 6, 12, 20, 30,………..are Pronic numbers.Using a switch statement, write a menu driven program to:
(a) generate and display the first 10 terms of the Fibonacci series
0, 1, 1, 2, 3, 5
The first two Fibonacci numbers are 0 and 1, and each subsequent number is the sum of the previous two.(b) find the sum of the digits of an integer that is input by the user.
Sample Input: 15390
Sample Output: Sum of the digits = 18
For an incorrect choice, an appropriate error message should be displayed.Using switch statement, write a menu driven program for the following:
(a) To find and display the sum of the series given below:
S = x1 - x2 + x3 - x4 + x5 - ………………….. - x20
(b) To find and display the sum of the series given below:
S = ………………….. to n terms
For an incorrect option, an appropriate error message should be displayed.