Computer Applications
Write the program to find the sum of the following series:
S = a + a2 + a3 + ……. + an
Java
Java Iterative Stmts
47 Likes
Answer
import java.util.Scanner;
public class KboatSeries
{
public void computeSeriesSum() {
Scanner in = new Scanner(System.in);
System.out.print("Enter a: ");
int a = in.nextInt();
System.out.print("Enter n: ");
int n = in.nextInt();
int sum = 0;
for (int i = 1; i <= n; i++) {
sum += Math.pow(a, i);
}
System.out.println("Sum=" + sum);
}
}
Variable Description Table
Program Explanation
Output
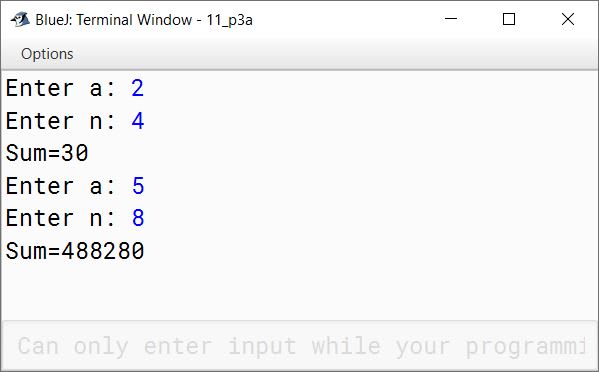
Answered By
19 Likes
Related Questions
Write the program in Java to find the sum of the following series:
S = 1 + (1*2) + (1*2*3) + ……. + (1*2*3* ……. * n)
Write the program in Java to find the sum of the following series:
S = 1 + (1 + 2) / (1 * 2) + (1 + 2 + 3) / (1 * 2 * 3) + ……. + (1 + 2 + 3 ……. + n) / (1 * 2 * 3 * ……. * n)
Write the program to find the sum of the following series:
S = (a+1) + (a+2) + (a+3) + ……. + (a+n)
Write the program to find the sum of the following series:
S = (a/2) + (a/5) + (a/8) + (a/11) + ……. + (a/20)