Computer Applications
Write Java program to find the sum of the given series:
1 + (1+2) + (1+2+3) + ………. + (1+2+3+ …… + n)
Java
Java Nested for Loops
18 Likes
Answer
import java.util.Scanner;
public class KboatSeriesSum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter n: ");
int n = in.nextInt();
long sum = 0;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
sum += j;
}
}
System.out.println("Sum=" + sum);
}
}
Variable Description Table
Program Explanation
Output
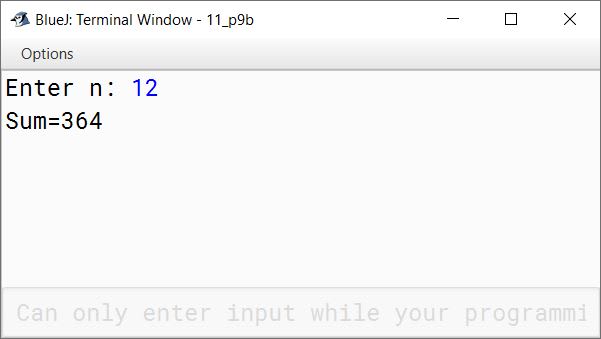
Answered By
10 Likes
Related Questions
Write Java program to find the sum of the given series:
1 + (1/2!) + (1/3!) + (1/4!) + ………. + (1/n!)
Write Java program to find the sum of the given series:
1 + 1 / (1+2) + 1 / (1+2+3) + ………. + 1 / (1+2+3+…..+n)
Write a program to input a number and perform the following tasks:
(a) to check whether it is a prime number or not
(b) to reverse the number
If the number as well as the reverse is also 'Prime' then display 'Twisted Prime' otherwise 'Not a twisted Prime'.
Sample Input: 167
Sample Output: 167 and 761 both are prime.
It is a 'Twisted Prime'.Write Java program to find the sum of the given series:
1 + (1*2) + (1*2*3) + ………. + (1*2*3* …… * n)