Computer Applications
Write a program to store 20 numbers in a Single Dimensional Array (SDA). Now, display only those numbers that are perfect squares.
n[0] | n[1] | n[2] | n[3] | n[4] | n[5] | … | n[16] | n[17] | n[18] | n[19] |
---|---|---|---|---|---|---|---|---|---|---|
12 | 45 | 49 | 78 | 64 | 77 | … | 81 | 99 | 45 | 33 |
Sample Output: 49, 64, 81
Java
Java Arrays
79 Likes
Answer
import java.util.Scanner;
public class KboatSDASquares
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[] = new int[20];
System.out.println("Enter 20 numbers");
for (int i = 0; i < arr.length; i++) {
arr[i] = in.nextInt();
}
System.out.println("Perfect Squares are:");
for (int i = 0; i < arr.length; i++) {
double sr = Math.sqrt(arr[i]);
if ((sr - Math.floor(sr)) == 0)
System.out.print(arr[i] + ", ");
}
}
}
Variable Description Table
Program Explanation
Output
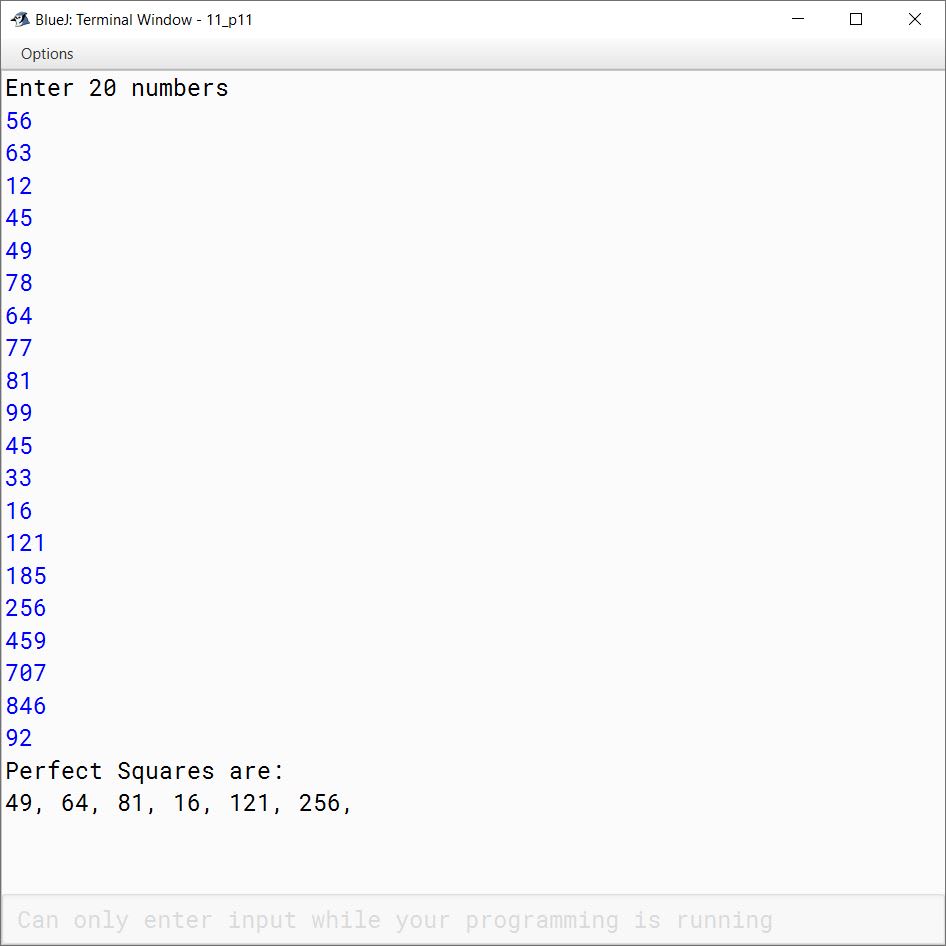
Answered By
31 Likes
Related Questions
The annual examination result of 50 students in a class is tabulated in a Single Dimensional Array (SDA) is as follows:
Roll No. Subject A Subject B Subject C ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. ……. Write a program to read the data, calculate and display the following:
(a) Average marks obtained by each student.
(b) Print the roll number and the average marks of the students whose average is above. 80.
(c) Print the roll number and the average marks of the students whose average is below 40.Write a program in Java to accept 20 numbers in a single dimensional array arr[20]. Transfer and store all the even numbers in an array even[ ] and all the odd numbers in another array odd[ ]. Finally, print the elements of both the arrays.
Write a program to accept a list of 20 integers. Sort the first 10 numbers in ascending order and next the 10 numbers in descending order by using 'Bubble Sort' technique. Finally, print the complete list of integers.
To get promotion in a Science stream, a student must pass in English and should pass in any of the two subjects (i.e.; Physics, Chemistry or Maths). The passing mark in each subject is 35. Write a program in a Single Dimension Array to accept the roll numbers and marks secured in the subjects for all the students. The program should check and display the roll numbers along with a message whether "Promotion is Granted" or "Promotion is not Granted". Assume that there are 40 students in the class.