Computer Applications
Write a program to input year and check whether it is:
(a) a Leap year
(b) a Century Leap year
(c) a Century year but not a Leap year
Sample Input: 2000
Sample Output: It is a Century Leap Year.
Java
Java Conditional Stmts
186 Likes
Answer
import java.util.Scanner;
public class KboatCenturyLeapYear
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the year to check: ");
int yr = in.nextInt();
if (yr % 4 == 0 && yr % 100 != 0)
System.out.println("It is a Leap Year");
else if (yr % 400 == 0)
System.out.println("It is a Century Leap Year");
else if (yr % 100 == 0)
System.out.println("It is a Century Year but not a Leap Year");
else
System.out.println("It is neither a Century Year nor a Leap Year");
}
}
Variable Description Table
Program Explanation
Output
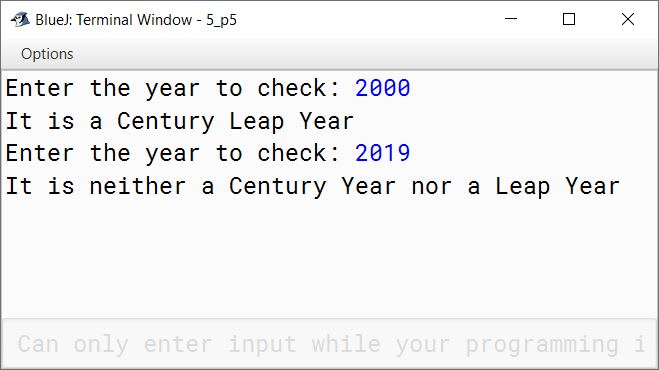
Answered By
66 Likes
Related Questions
Write a program to input three numbers and check whether they are equal or not. If they are unequal numbers then display the greatest among them otherwise, display the message 'All the numbers are equal'.
Sample Input: 34, 87, 61
Sample Output: Greatest number: 87
Sample Input: 81, 81, 81
Sample Output: All the numbers are equal.Write a program to accept a number and check whether the number is divisible by 3 as well as 5. Otherwise, decide:
(a) Is the number divisible by 3 and not by 5?
(b) Is the number divisible by 5 and not by 3?
(c) Is the number neither divisible by 3 nor by 5?
The program displays the message accordingly.
Write a program to input two unequal positive numbers and check whether they are perfect square numbers or not. If the user enters a negative number then the program displays the message 'Square root of a negative number can't be determined'.
Sample Input: 81, 100
Sample Output: They are perfect square numbers.
Sample Input: 225, 99
Sample Output: 225 is a perfect square number.
99 is not a perfect square number.Without using if-else statement and ternary operators, accept three unequal numbers and display the second smallest number.
[Hint: Use Math.max( ) and Math.min( )]
Sample Input: 34, 82, 61
Sample Output: 61