Computer Applications
Write a program to input two unequal numbers. Display the numbers after swapping their values in the variables without using a third variable.
Sample Input: a = 23, b = 56
Sample Output: a = 56, b = 23
Java
Input in Java
231 Likes
Answer
import java.util.Scanner;
public class KboatNumberSwap
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter two unequal numbers");
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
a = a + b;
b = a - b;
a = a - b;
System.out.println("a = " + a + " b = " + b);
}
}
Variable Description Table
Program Explanation
Output
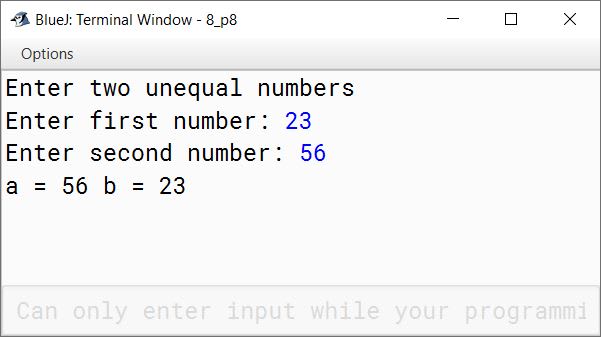
Answered By
93 Likes
Related Questions
A shopkeeper sells two calculators for the same price. He earns 20% profit on one and suffers a loss of 20% on the other. Write a program to find his total cost price of the calculators by taking selling price as input.
Hint: CP = (SP / (1 + (profit / 100))) (when profit)
CP = (SP / (1 - (loss / 100))) (when loss)A certain amount is invested at the rate 10% per annum for 3 years. Find the difference between Compound Interest (CI) and Simple Interest (SI). Write a program to take amount as an input.
Hint: SI = (P * R * T) / 100
A = P * (1 + (R/100))T
CI = A - PWrite a program to input time in seconds. Display the time after converting them into hours, minutes and seconds.
Sample Input: Time in seconds: 5420
Sample Output: 1 Hour 30 Minutes 20 Seconds
A businessman wishes to accumulate 3000 shares of a company. However, he already has some shares of that company valuing ₹10 (nominal value) which yield 10% dividend per annum and receive ₹2000 as dividend at the end of the year. Write a program in Java to calculate the number of shares he has and how many more shares to be purchased to make his target.
Hint: No. of share = (Annual dividend * 100) / (Nominal value * div%)