Computer Applications
Write a program to input three unequal numbers. Display the greatest and the smallest number.
Sample Input: 28, 98, 56
Sample Output: Greatest Number: 98
Smallest Number: 28
Java
Java Conditional Stmts
143 Likes
Answer
import java.util.Scanner;
public class KboatMinMaxNumbers
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter 3 unequal numbers");
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
System.out.print("Enter third number: ");
int c = in.nextInt();
int min = a, max = a;
min = b < min ? b : min;
min = c < min ? c : min;
max = b > max ? b : max;
max = c > max ? c : max;
System.out.println("Greatest Number: " + max);
System.out.println("Smallest Number: " + min);
}
}
Variable Description Table
Program Explanation
Output
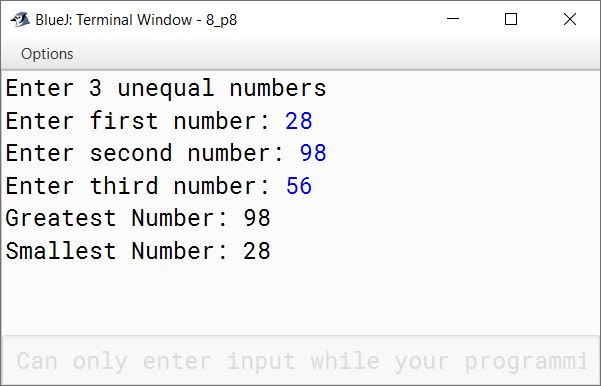
Answered By
54 Likes
Related Questions
Without using if-else statement and ternary operators, accept three unequal numbers and display the second smallest number.
[Hint: Use Math.max( ) and Math.min( )]
Sample Input: 34, 82, 61
Sample Output: 61Write a program to input two unequal positive numbers and check whether they are perfect square numbers or not. If the user enters a negative number then the program displays the message 'Square root of a negative number can't be determined'.
Sample Input: 81, 100
Sample Output: They are perfect square numbers.
Sample Input: 225, 99
Sample Output: 225 is a perfect square number.
99 is not a perfect square number.A cloth showroom has announced festival discounts and the gifts on the purchase of items, based on the total cost as given below:
Total Cost Discount Gift Up to ₹ 2,000 5% Calculator ₹ 2,001 to ₹ 5,000 10% School Bag ₹ 5,001 to ₹ 10,000 15% Wall Clock Above ₹ 10,000 20% Wrist Watch Write a program to input the total cost. Compute and display the amount to be paid by the customer along with the gift.
A Pre-Paid taxi charges from the passenger as per the tariff given below:
Distance Rate Up to 5 km ₹ 100 For the next 10 km ₹ 10/km For the next 10 km ₹ 8/km More than 25 km ₹ 5/km Write a program to input the distance covered and calculate the amount paid by the passenger. The program displays the printed bill with the details given below:
Taxi No. :
Distance covered :
Amount :