Computer Applications
Write a program to input three numbers (positive or negative). If they are unequal then display the greatest number otherwise, display they are equal. The program also displays whether the numbers entered by the user are 'All positive', 'All negative' or 'Mixed numbers'.
Sample Input: 56, -15, 12
Sample Output:
The greatest number is 56
Entered numbers are mixed numbers.
Java
Java Conditional Stmts
131 Likes
Answer
import java.util.Scanner;
public class KboatNumberAnalysis
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
System.out.print("Enter third number: ");
int c = in.nextInt();
int greatestNumber = a;
if ((a == b) && (b == c)) {
System.out.println("Entered numbers are equal.");
}
else {
if (b > greatestNumber) {
greatestNumber = b;
}
if (c > greatestNumber) {
greatestNumber = c;
}
System.out.println("The greatest number is " + greatestNumber);
if ((a >= 0) && (b >= 0) && (c >= 0)) {
System.out.println("Entered numbers are all positive numbers.");
}
else if((a < 0) && (b < 0) && (c < 0)) {
System.out.println("Entered numbers are all negative numbers.");
}
else {
System.out.println("Entered numbers are mixed numbers.");
}
}
}
}
Variable Description Table
Program Explanation
Output
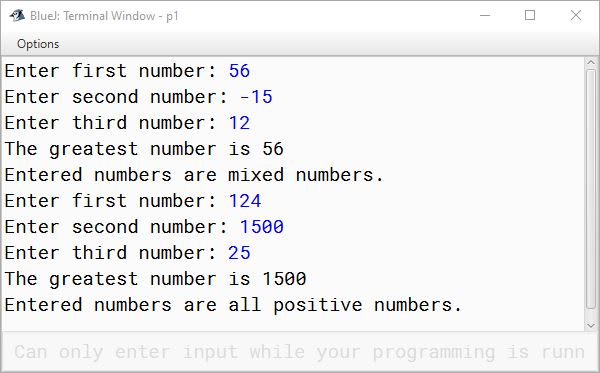
Answered By
51 Likes
Related Questions
An air-conditioned bus charges fare from the passengers based on the distance travelled as per the tariff given below:
Distance Travelled Fare Up to 10 km Fixed charge ₹80 11 km to 20 km ₹6/km 21 km to 30 km ₹5/km 31 km and above ₹4/km Design a program to input distance travelled by the passenger. Calculate and display the fare to be paid.
A special two-digit number is such that when the sum of its digits is added to the product of its digits, the result is equal to the original two-digit number.
Example: Consider the number 59.
Sum of digits = 5 + 9 = 14
Product of digits = 5 * 9 = 45
Total of the sum of digits and product of digits = 14 + 45 = 59
Write a program to accept a two-digit number. Add the sum of its digits to the product of its digits. If the value is equal to the number input, display the message "Special 2 - digit number" otherwise, display the message "Not a special two-digit number".A triangle is said to be an 'Equable Triangle', if the area of the triangle is equal to its perimeter. Write a program to enter three sides of a triangle. Check and print whether the triangle is equable or not.
For example, a right angled triangle with sides 5, 12 and 13 has its area and perimeter both equal to 30.The standard form of quadratic equation is given by: ax2 + bx + c = 0, where d = b2 - 4ac, is known as discriminant that determines the nature of the roots of the equation as:
Condition Nature if d >= 0 Roots are real if d < 0 Roots are imaginary Write a program to determine the nature and the roots of a quadratic equation, taking a, b, c as input. If d = b2 - 4ac is greater than or equal to zero, then display 'Roots are real', otherwise display 'Roots are imaginary'. The roots are determined by the formula as:
r1 = (-b + √(b2 - 4ac)) / 2a , r2 = (-b - √(b2 - 4ac)) / 2a