Computer Applications
Write a program to input three numbers and print the largest of the three numbers.
Java
Java Conditional Stmts
7 Likes
Answer
import java.util.Scanner;
public class KboatLargestNumber
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int a = in.nextInt();
System.out.print("Enter second number: ");
int b = in.nextInt();
System.out.print("Enter third number: ");
int c = in.nextInt();
System.out.print("Largest number: ");
if (a > b && a > c)
System.out.println(a);
else if (b > a && b > c)
System.out.println(b);
else
System.out.println(c);
}
}
Variable Description Table
Program Explanation
Output
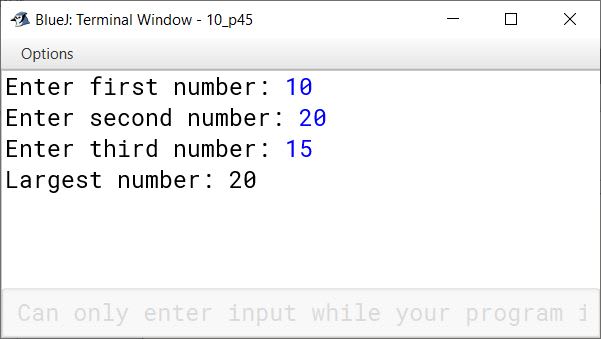
Answered By
3 Likes
Related Questions
Write a program that inputs an alphabet and checks if the given alphabet is a vowel or not.
Write a program that prints the squares of 10 even numbers in the range 10 .. 100.
Write a program that takes a number and check if the given number is a 3 digit number or not. (Use if to determine)
Write a program that takes a number and check if the given number is a 3 digit number or not. (Use a loop to determine)