Computer Science
Write a program to input N and M number of names in two different single dimensional arrays A and B respectively, such that none of them have duplicate names. Merge the arrays A and B into a single array C, such that the resulting array is sorted alphabetically. Display all the three arrays.
Test your program for the following data and some random data:
Sample data:
Input:
Enter the names in array A, N = 2
Enter the names in array B, M = 3
First array: A
Suman
Anil
Second array: B
Usha
Sachin
John
Output:
Sorted Merged array: C
Anil
John
Sachin
Suman
Usha
Sorted First array: A
Anil
Suman
Sorted Second array: B
John
Sachin
Usha
Java
Java Arrays
7 Likes
Answer
import java.util.Scanner;
public class KboatSDANames
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the names in array A, N = ");
int n = in.nextInt();
System.out.print("Enter the names in array B, M = ");
int m = in.nextInt();
in.nextLine();
String a[] = new String[n];
String b[] = new String[m];
String c[] = new String[m+n];
System.out.println("First array: A");
for (int i = 0; i < n; i++) {
String name = in.nextLine();
for (int j = i - 1; j >= 0; j--) {
if (a[j].equalsIgnoreCase(name)) {
System.out.println(name + " already present in array");
return;
}
}
a[i] = name;
}
System.out.println("Second array: B");
for (int i = 0; i < m; i++) {
String name = in.nextLine();
for (int k = 0; k < n; k++) {
if (a[k].equalsIgnoreCase(name)) {
System.out.println(name + " already present in array");
return;
}
}
for (int j = i - 1; j >= 0; j--) {
if (b[j].equalsIgnoreCase(name)) {
System.out.println(name + " already present in array");
return;
}
}
b[i] = name;
}
sortArray(a);
sortArray(b);
int aIdx = 0, bIdx = 0;
//Merge the arrays preserving the sorting
for (int i = 0; i < m+n; i++) {
if (aIdx == n || a[aIdx].compareToIgnoreCase(b[bIdx]) > 0) {
c[i] = b[bIdx];
bIdx++;
}
else if (bIdx == m || b[bIdx].compareToIgnoreCase(a[aIdx]) > 0) {
c[i] = a[aIdx];
aIdx++;
}
}
System.out.println("Sorted Merged array: C");
printArray(c);
System.out.println("Sorted First array: A");
printArray(a);
System.out.println("Sorted Second array: B");
printArray(b);
}
public static void sortArray(String arr[]) {
for (int i = 0; i < arr.length; i++) {
for (int j = 1; j < arr.length - i; j++) {
if (arr[j - 1].compareToIgnoreCase(arr[j]) > 0) {
String t = arr[j - 1];
arr[j - 1] = arr[j];
arr[j] = t;
}
}
}
}
public static void printArray(String arr[]) {
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
}
}
Output
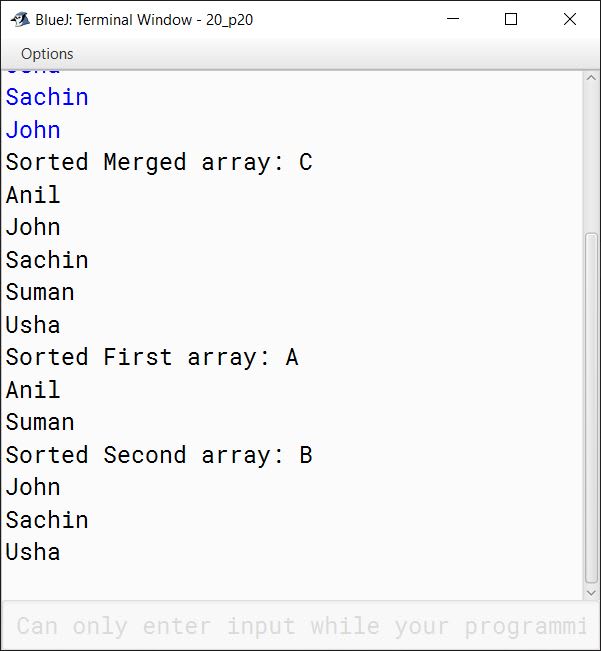
Answered By
3 Likes
Related Questions
Write a program in Java to store the elements in two different double dimensional arrays (in matrix form) A and B each of order 4 x 4. Find the product of both the matrices and store the result in matrix C. Display the elements of matrix C.
Note:
Two matrixes can be multiplied only if the number of columns of the first matrix must be equal to the number of rows of the second matrix.Sample Input: Matrix A
3 2 1 2 6 4 5 0 7 -1 0 2 4 3 1 1 Sample Input: Matrix B
-2 -4 -1 0 3 6 -5 2 5 3 4 6 0 -2 2 5 Sample Output: Matrix C
5 -1 -5 20 25 15 -6 38 -17 -38 2 -8 6 3 -13 17 - Write a program to declare a square matrix A[ ][ ] of order N (N<20).
- Allow the user to input positive integers into this matrix. Perform the following tasks on the matrix.
- Output the original matrix.
- Find the SADDLE POINT for the matrix. A saddle point is an element of the matrix such that it is the minimum element for the row and the maximum element for the column to which it belongs. Saddle point for a given matrix is always unique. If the matrix has no saddle point, output the message "NO SADDLE POINT".
- If the matrix has a saddle point element then sort the elements of the left diagonal in ascending order using insertion sort technique. All other elements should remain unchanged.
Test your program for the following data and some random data:
Sample data:
Input:
n = 4Matrix A[ ][ ] 2 5 6 9 8 4 12 3 6 7 3 1 12 24 2 11 Output:
Matrix A[ ][ ] 2 5 6 9 8 4 12 3 6 7 3 1 12 24 2 11 No Saddle Point
Matrix after sorting the Principal diagonal:
2 5 6 9 8 3 12 3 6 7 4 1 12 24 2 11 Input:
n = 3Matrix A[ ][ ] 4 6 12 2 8 14 1 3 6 Output:
Matrix A[ ][ ] 4 6 12 2 8 14 1 3 6 Saddle Point = 4
Matrix after sorting the Principal diagonal:
4 6 12 2 6 14 1 3 8 Numbers have different representations depending on the bases on which they are expressed. For example, in base 3, the number 12 is written as 110 (1 x 32 + 1 x 31 + 0 x 30) but base 8 it is written as 14 (1 x 81 + 4 x 80).
Consider for example, the integers 12 and 5. Certainly these are not equal if base 10 is used for each. But suppose, 12 was a base 3 number and 5 was a base 6 number then, 12 base 3 = 1 x 31 + 2 x 30, or 5 base 6 or base 10 (5 in any base is equal to 5 base 10). So, 12 and 5 can be equal if you select the right bases for each of them.
Write a program to input two integers x and y and calculate the smallest base for x and smallest base for y (likely different from x) so that x and y represent the same value. The base associated with x and y will be between 1 and 20 (both inclusive). In representing these numbers, the digits 0 to 9 have their usual decimal interpretations. The upper case letters from A to J represent digits 10 to 19 respectively.
Test your program for the following data and some random data.
Sample Data
Input:
x=12, y=5Output:
12 (base 3)=5 (base 6)Input:
x=10, y=AOutput:
10 (base 10)=A (base 11)Input:
x=12, y=34Output:
~~12 (base 8) = 34 (base 2)~~
12 (base 17) = 34 (base 5)
[∵ 34 (base 2) is not valid as only 0 & 1 are allowed in base 2]Input:
x=123, y=456Output:
123 is not equal to 456 in any base between 2 to 20Input:
x=42, y=36Output:
42 (base 7) = 36 (base 8)The manager of a company wants to analyze the machine usage from the records to find the utilization of the machine. He wants to know how long each user used the machine. When the user wants to use the machine, he must login to the machine and after finishing the work, he must logoff the machine.
Each log record consists of:
User Identification number
Login time and date
Logout time and dateTime consists of:
Hours
MinutesDate consists of:
Day
MonthYou may assume all logins and logouts are in the same year and there are 100 users at the most. The time format is 24 hours.
Design a program:
(a) To find the duration for which each user logged. Output all records along with the duration in hours (format hours: minutes).
(b) Output the record of the user who logged for the longest duration. You may assume that no user will login for more than 48 hours.
Test your program for the following data and some random data.
Sample Data
Input:
Number of users: 3
User IdentificationLogin Time and Date Logout Time and Date 20:10 20-12 2:50 21-12 12:30 20-12 12:30 21-12 16:20 20-12 16:30 20-12 Output:
User Identification Login Time and Date Logout Time and Date Duration
Hours:Minutes149 20:10 20-12 2:50 21-12 6:40 173 12:30 20-12 12:30 21-12 24:00 142 16:20 20-12 16:30 20-12 00:10 The user who logged in for longest duration:
173 12:30 20-12 12:30 21-12 24:00